在Android中使用Java语言 运用EasyDL结合OpenCV 实现在图片中查找目标物体
时间: 2024-02-26 20:57:49 浏览: 26
在 Android 中使用 EasyDL 结合 OpenCV 实现在图片中查找目标物体,可以按照以下步骤进行:
1. 在 EasyDL 平台上创建一个物体检测模型,并部署到 EasyDL SDK 上。
2. 在 Android 项目中添加 EasyDL SDK 和 OpenCV 的依赖项。在 build.gradle 文件中添加以下依赖项:
```
dependencies {
implementation 'com.baidu.ai:easydl-sdk:1.0.1'
implementation 'org.opencv:opencv-android:3.4.3'
}
```
3. 在代码中加载模型,并使用模型进行物体检测。以下是一个简单的示例代码:
```
// 加载 EasyDL 模型
EasyDLClient client = new EasyDLClient("<your model id>", "<your api key>");
// 加载 OpenCV 图像
Mat image = Imgcodecs.imread("<your image path>");
// 使用 EasyDL 模型进行物体检测
EasyDLResult result = client.predict(image);
// 解析检测结果
List<Object> outputs = result.getOutputs();
for (Object output : outputs) {
// 处理检测结果
}
```
在上面的代码中,`<your model id>` 和 `<your api key>` 是在 EasyDL 平台上创建的模型 ID 和 API Key,`<your image path>` 是需要进行物体检测的图片路径。
4. 处理检测结果,并在图片中标记出目标物体的位置。以下是一个简单的示例代码:
```
// 获取检测结果
List<Object> outputs = result.getOutputs();
// 遍历检测结果
for (Object output : outputs) {
if (output instanceof DetectionOutput) {
DetectionOutput detectionOutput = (DetectionOutput) output;
List<DetectionOutput.BBox> bBoxes = detectionOutput.getBboxes();
for (DetectionOutput.BBox bbox : bBoxes) {
// 获取物体位置信息
int x1 = (int) (bbox.getX1() * image.width());
int y1 = (int) (bbox.getY1() * image.height());
int x2 = (int) (bbox.getX2() * image.width());
int y2 = (int) (bbox.getY2() * image.height());
// 在图片上标记出物体位置
Imgproc.rectangle(image, new Point(x1, y1), new Point(x2, y2), new Scalar(0, 0, 255), 2);
}
}
}
```
在上面的代码中,我们首先判断输出是否是 DetectionOutput 类型,然后获取其中的物体位置信息,并使用 OpenCV 的 rectangle 方法在图片上标记出物体的位置。
注意:在使用 OpenCV 的方法时,需要在代码中加载 OpenCV 的本地库。具体可以在 build.gradle 文件中添加以下配置:
```
android {
// ...
defaultConfig {
// ...
ndk {
// ...
abiFilters 'armeabi-v7a', 'arm64-v8a', 'x86', 'x86_64'
}
}
// ...
}
// 加载 OpenCV 的本地库
static {
System.loadLibrary("opencv_java3");
}
```
相关推荐
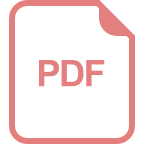
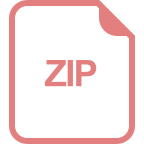















