c++在指定文件夹中根据时间创建文件夹,文件夹名为当前的时间,格式为:%Y-%m-%d-%H-%M每10min中生成一个新的,文件夹命名时间分钟必须为十的倍数,同时再该文件夹下再创建一个文件夹
时间: 2023-08-17 12:04:20 浏览: 66
可以使用C++中的ctime和chrono库来获取当前时间和延时等操作来实现您的需求。以下是一个简单的示例代码:
```cpp
#include <iostream>
#include <ctime>
#include <chrono>
#include <string>
#include <windows.h>
using namespace std;
int main() {
while (true) {
auto now = chrono::system_clock::now();
auto now_c = chrono::system_clock::to_time_t(now);
struct tm* parts = localtime(&now_c);
// 判断时间是否为10的倍数
if (parts->tm_min % 10 != 0) {
Sleep(600000); // 如果不是10的倍数则延时10min
continue;
}
// 创建文件夹
string folder_name = to_string(parts->tm_year + 1900) + "-"
+ to_string(parts->tm_mon + 1) + "-"
+ to_string(parts->tm_mday) + "-"
+ to_string(parts->tm_hour) + "-"
+ to_string(parts->tm_min);
string folder_path = "C:\\your\\folder\\path\\" + folder_name;
if (CreateDirectory(folder_path.c_str(), NULL) ||
ERROR_ALREADY_EXISTS == GetLastError()) {
// 创建成功或者已经存在
string sub_folder_path = folder_path + "\\sub_folder";
if (CreateDirectory(sub_folder_path.c_str(), NULL) ||
ERROR_ALREADY_EXISTS == GetLastError()) {
// 创建成功或者已经存在
cout << "创建文件夹成功:" << sub_folder_path << endl;
}
else {
cerr << "创建子文件夹失败!" << endl;
}
}
else {
cerr << "创建文件夹失败!" << endl;
}
Sleep(600000); // 延时10min
}
return 0;
}
```
注意:该代码仅为示例,实际应用中需要根据实际情况进行修改和完善。
相关推荐
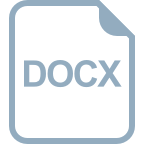
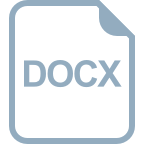
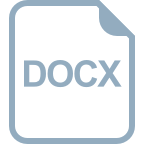















