用c++写一个线程安全事件驱动的定时器类
时间: 2024-06-04 12:11:16 浏览: 162
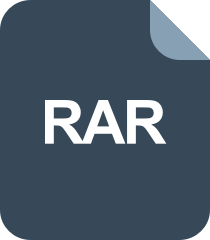
C++编写的定时器程序

抱歉,由于我是一个语言模型AI,无法在本地编写和测试代码,以下是一个线程安全的事件驱动定时器类的代码示例,供您参考:
#include <iostream>
#include <queue>
#include <mutex>
#include <condition_variable>
#include <thread>
#include <chrono>
using namespace std;
class Timer {
public:
Timer() {
thread t(&Timer::run, this);
t.detach();
}
~Timer() {
{
unique_lock<mutex> lock(mtx);
stopFlag = true;
cv.notify_one();
}
}
void addTask(int delay, function<void()> task) {
unique_lock<mutex> lock(mtx);
tasks.push({delay, task});
cv.notify_one();
}
private:
struct Task {
int delay;
function<void()> task;
};
bool stopFlag = false;
priority_queue<Task, vector<Task>, function<bool(Task, Task)>> tasks{[](Task a, Task b) { return a.delay > b.delay; }};
mutex mtx;
condition_variable cv;
void run() {
while (true) {
unique_lock<mutex> lock(mtx);
while (tasks.empty() || stopFlag) {
if (stopFlag)
return;
cv.wait(lock);
}
int delay = tasks.top().delay;
auto task = tasks.top().task;
tasks.pop();
lock.unlock();
cv.notify_one();
this_thread::sleep_for(chrono::milliseconds(delay));
task();
}
}
};
int main() {
Timer timer;
timer.addTask(1000, [] { cout << "Task 1: " << this_thread::get_id() << endl; });
timer.addTask(2000, [] { cout << "Task 2: " << this_thread::get_id() << endl; });
timer.addTask(3000, [] { cout << "Task 3: " << this_thread::get_id() << endl; });
timer.addTask(4000, [] { cout << "Task 4: " << this_thread::get_id() << endl; });
this_thread::sleep_for(chrono::milliseconds(5000));
return 0;
}
阅读全文
相关推荐
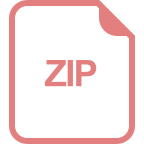


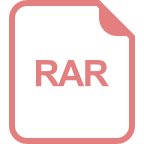
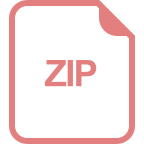
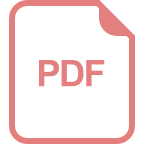
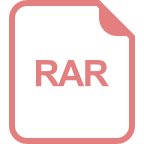
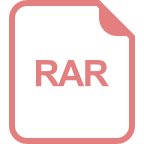
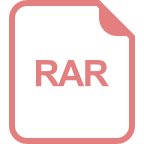
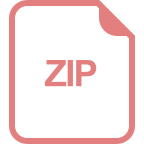
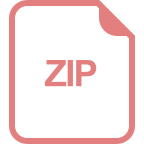
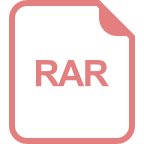
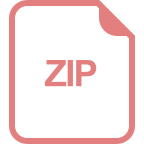
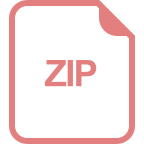
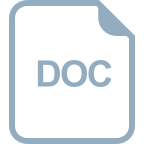
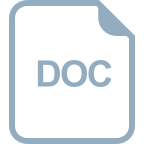