# 执行 PowerShell 命令 process = subprocess.Popen(['powershell.exe', '-ExecutionPolicy', 'Bypass', '-Command',powershell_script], stdout=subprocess.PIPE, stderr=subprocess.PIPE) process.stdout.read().decode("utf-8")出现'utf-8' codec can't decode byte 0xd5 in position 16: invalid continuation byte怎么解决
时间: 2023-12-21 10:06:46 浏览: 39
这个错误是因为 `decode()` 方法无法解码某些字节序列。在这种情况下,你可以尝试使用其他的解码方式,例如 'gbk' 或 'utf-16' 等。
你可以修改代码,将 `decode("utf-8")` 改为其他编码方式,例如:
```python
process.stdout.read().decode("gbk")
```
如果你不确定使用哪种编码方式,可以尝试使用 Python 的 `chardet` 模块来自动检测编码方式。你可以将以下代码插入到你的程序中:
```python
import chardet
result = chardet.detect(process.stdout.read())
print(result['encoding'])
```
这段代码会自动检测编码方式并输出结果。然后你可以将输出的编码方式作为参数传递给 `decode()` 方法,例如:
```python
process.stdout.read().decode(result['encoding'])
```
相关问题
process = subprocess.Popen(['powershell.exe', '-ExecutionPolicy', 'Bypass', '-Command',\ powershell_script], stdout=subprocess.PIPE, stderr=subprocess.PIPE)
这是一个使用Python的subprocess模块执行PowerShell脚本的代码示例。其中,'powershell.exe'指定了要执行的命令为PowerShell,'-ExecutionPolicy'指定了Bypass参数以跳过执行策略,'-Command'后面跟着要执行的PowerShell脚本。执行结果会被输出到stdout和stderr中。
def mac_code_confirm(): import subprocess # 定义 PowerShell 命令 ps_command = r'Get-WmiObject Win32_ComputerSystemProduct | Select-Object -ExpandProperty UUID' # 执行 PowerShell 命令并获取输出 result = subprocess.run(['powershell', '-Command', ps_command], capture_output=True, text=True) # 检查 PowerShell 命令是否执行成功 if result.returncode == 0: machine_code = result.stdout.strip() expected_machine_code = "9E19AA0D-772D-C93B-3544-50EBF6592F65" if machine_code != expected_machine_code: print("未授权") import subprocess exit()优化这段代码
这段代码的作用是获取计算机的唯一标识符,并与预期的标识符进行比较,如果不匹配则输出未授权并退出程序。
可以对代码进行如下优化:
1. 使用 f-string 格式化字符串,可以使代码更加简洁、易读。
2. 使用 subprocess 的 check_output 方法,可以在命令执行失败时抛出异常。
3. 退出程序可以直接使用 sys 模块的 exit 函数,不需要使用 subprocess 调用 exit 命令。
优化后的代码如下所示:
```
import subprocess
import sys
def mac_code_confirm():
# 定义 PowerShell 命令
ps_command = r'Get-WmiObject Win32_ComputerSystemProduct | Select-Object -ExpandProperty UUID'
# 执行 PowerShell 命令并获取输出
try:
machine_code = subprocess.check_output(['powershell', '-Command', ps_command], text=True).strip()
except subprocess.CalledProcessError:
print("获取计算机唯一标识符失败")
sys.exit(1)
expected_machine_code = "9E19AA0D-772D-C93B-3544-50EBF6592F65"
if machine_code != expected_machine_code:
print("未授权")
sys.exit(1)
```
这样可以使代码更加简洁、易读,并且在错误处理上也更加健壮。
相关推荐
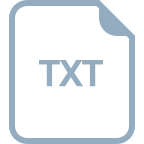
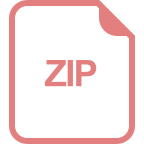













