用Python通过遗传算法求函数F(x)=xsin(10π*x)+2.0 x∈[-1,2]的最大值。
时间: 2023-10-07 14:13:24 浏览: 122
好的,下面是一个简单的Python代码实现,使用遗传算法求解函数F(x)的最大值:
```python
import random
import math
# 定义函数F(x)
def F(x):
return x * math.sin(10 * math.pi * x) + 2.0
# 定义遗传算法参数
POP_SIZE = 50 # 种群大小
GENE_LENGTH = 20 # 基因长度
CROSS_RATE = 0.8 # 交叉概率
MUTATE_RATE = 0.01 # 变异概率
N_GENERATIONS = 200 # 迭代次数
# 初始化种群
pop = [[random.uniform(-1, 2) for _ in range(GENE_LENGTH)] for _ in range(POP_SIZE)]
# 遗传算法迭代
for generation in range(N_GENERATIONS):
# 计算每个个体的适应度
fitness = [F(ind) for ind in pop]
# 选择优秀个体作为父代
parents = []
for _ in range(POP_SIZE):
idx1, idx2 = random.randint(0, POP_SIZE-1), random.randint(0, POP_SIZE-1)
if fitness[idx1] > fitness[idx2]:
parents.append(pop[idx1])
else:
parents.append(pop[idx2])
# 交叉操作
for child in parents:
if random.random() < CROSS_RATE:
idx = random.randint(0, POP_SIZE-1)
cross_points = random.randint(0, GENE_LENGTH)
child[cross_points:] = pop[idx][cross_points:]
# 变异操作
for child in parents:
if random.random() < MUTATE_RATE:
mutate_point = random.randint(0, GENE_LENGTH-1)
child[mutate_point] += random.uniform(-0.1, 0.1)
# 更新种群
pop = parents
# 输出最优解
best_ind = pop[0]
best_fitness = F(best_ind)
for ind in pop:
if F(ind) > best_fitness:
best_ind = ind
best_fitness = F(ind)
print("最优解:", best_ind)
print("最大值:", best_fitness)
```
这段代码使用了Python的随机数生成函数,以及math库中的数学函数。遗传算法部分的实现也比较简单,主要包括种群初始化、适应度计算、选择、交叉、变异和种群更新等步骤。通过迭代,可以逐步逼近函数F(x)的最大值。
阅读全文
相关推荐
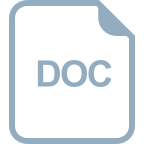
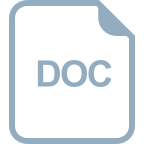
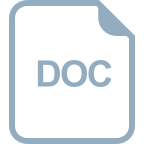










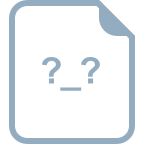


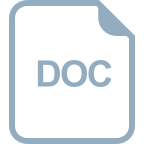