C语言实现程序读取一段字符,统计出现概率,并以此为权值创建哈夫曼树并以此为进行编码解码
时间: 2024-05-02 15:22:52 浏览: 92
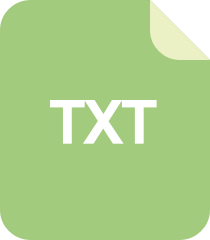
哈夫曼树的c语言实现

好的,我会为您解答这个问题。首先,我们需要对输入的字符串进行统计,计算每个字符出现的概率。
可以创建一个结构体来存储每个字符和它出现的次数,比如:
```c
struct node {
char symbol;
int frequency;
};
```
然后,我们可以使用数组来存储所有的结构体,同时记录下字符的总数。具体的代码如下所示:
```c
char input_string[MAX_SIZE]; // 定义输入字符串
struct node freq_table[MAX_SIZE]; // 定义结构体数组
int char_count = 0; // 字符计数器
int freq_table_size = 0; // 频率表大小
// 读取输入字符串,并进行字符计数
scanf("%s", input_string);
for (int i = 0; i < strlen(input_string); i++) {
char_count++;
bool found = false;
for (int j = 0; j < freq_table_size; j++) {
if (freq_table[j].symbol == input_string[i]) {
freq_table[j].frequency++;
found = true;
break;
}
}
if (!found) {
freq_table[freq_table_size].symbol = input_string[i];
freq_table[freq_table_size].frequency = 1;
freq_table_size++;
}
}
```
接下来,我们根据字符的出现概率来创建哈夫曼树。首先,我们需要根据字符的频率构建一个节点结构体。节点结构体包含了字符、频率、左右子节点等信息。比如:
```c
struct node {
char symbol;
int frequency;
struct node *left;
struct node *right;
};
```
然后,我们可以使用一个优先队列(堆)来存储所有的节点,按照节点的频率排序。具体的代码如下所示:
```c
// 定义优先队列和队列大小
struct node *pq[MAX_SIZE];
int pq_size = 0;
// 将所有节点插入优先队列
for (int i = 0; i < freq_table_size; i++) {
struct node *n = (struct node *) malloc(sizeof(struct node));
n->symbol = freq_table[i].symbol;
n->frequency = freq_table[i].frequency;
n->left = NULL;
n->right = NULL;
insert(pq, &pq_size, n);
}
// 通过循环构建哈夫曼树
while (pq_size > 1) {
struct node *n1 = pop(pq, &pq_size);
struct node *n2 = pop(pq, &pq_size);
struct node *parent = (struct node *) malloc(sizeof(struct node));
parent->symbol = '\0';
parent->frequency = n1->frequency + n2->frequency;
parent->left = n1;
parent->right = n2;
insert(pq, &pq_size, parent);
}
// 构建完毕后,优先队列中只剩下一个节点,即哈夫曼树的根节点
struct node *root = pop(pq, &pq_size);
```
最后,我们可以遍历哈夫曼树,给每个字符赋予对应的编码。编码可以用 0 和 1 来表示,比如 0 表示向左走,1 表示向右走。具体的代码如下所示:
```c
void traverse(struct node *node, char *path, int depth) {
if (node == NULL) return;
if (node->left == NULL && node->right == NULL) {
printf("%c: %s\n", node->symbol, path);
return;
}
path[depth] = '0';
traverse(node->left, path, depth + 1);
path[depth] = '1';
traverse(node->right, path, depth + 1);
}
// 执行遍历操作
char path[MAX_SIZE];
traverse(root, path, 0);
```
这样,就完成了 C 语言实现程序读取一段字符,统计出现概率,并以此为权值创建哈夫曼树并进行编码解码的过程。
阅读全文
相关推荐
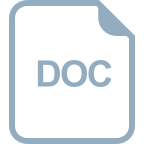
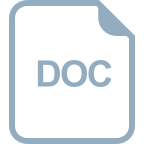
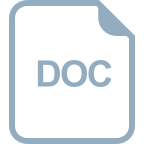
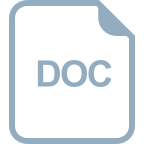
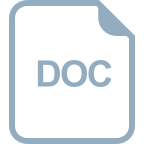









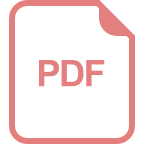
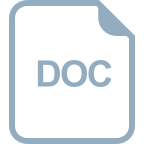
