Write a function is_bst, which takes a Tree t and returns True if, and only if, t is a valid binary search tree, which means that: Each node has at most two children (a leaf is automatically a valid binary search tree). The children are valid binary search trees. For every node, the entries in that node's left child are less than or equal to the label of the node. For every node, the entries in that node's right child are greater than the label of the node.其中class Tree: def init(self, label, branches=[]): for b in branches: assert isinstance(b, Tree) self.label = label self.branches = list(branches) def is_leaf(self): return not self.branches def map(self, fn): self.label = fn(self.label) for b in self.branches: b.map(fn) def contains(self, e): if self.label == e: return True for b in self.branches: if e in b: return True return False def repr(self): if self.branches: branch_str = ', ' + repr(self.branches) else: branch_str = '' return 'Tree({0}{1})'.format(self.label, branch_str) def str(self): def print_tree(t, indent=0): tree_str = ' ' * indent + str(t.label) + "\n" for b in t.branches: tree_str += print_tree(b, indent + 1) return tree_str return print_tree(self).rstrip()
时间: 2024-03-03 11:50:42 浏览: 100
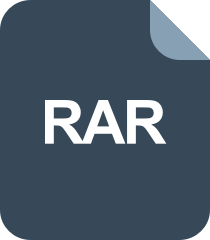
bst.rar_binary search tree_bst tree_in
Here is the implementation of the is_bst function in Python:
```
def is_bst(t):
def is_bst_helper(t, min_val, max_val):
if t.is_leaf():
return True
elif len(t.branches) == 1:
return False
else:
left = t.branches[0]
right = t.branches[1]
if left.label > t.label or right.label < t.label:
return False
if min_val is not None and left.label <= min_val:
return False
if max_val is not None and right.label >= max_val:
return False
return is_bst_helper(left, min_val, t.label) and is_bst_helper(right, t.label, max_val)
return is_bst_helper(t, None, None)
```
The is_bst function takes a Tree object as input and returns True if the tree is a valid binary search tree and False otherwise. The function uses a helper function, is_bst_helper, to recursively check if each node in the tree satisfies the conditions of a binary search tree.
The helper function takes three arguments: the current node, the minimum value that the entries in the left subtree can take, and the maximum value that the entries in the right subtree can take. If the current node is a leaf, the function returns True. If the current node has only one child or the entries in the left or right subtree violate the binary search tree property, the function returns False. Otherwise, the function recursively calls itself on the left and right subtrees, updating the minimum and maximum values as it goes.
Finally, the is_bst function calls the helper function on the root of the tree, with minimum and maximum values of None (since the root has no parent node), and returns the result of the helper function.
阅读全文
相关推荐
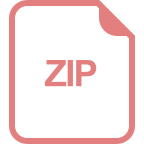
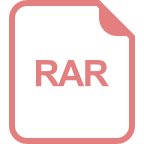







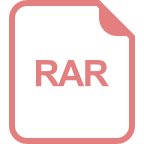
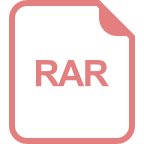
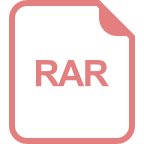
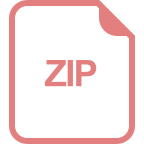
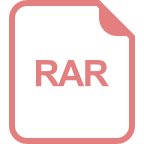
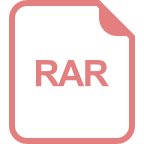
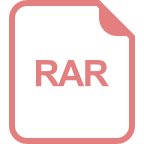
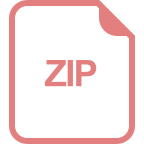