Python计算总成绩
时间: 2024-05-24 13:08:20 浏览: 32
计算Python课程总成绩的方法可以按照以下步骤进行:
1. 设定每个考核部分的权重,比如期末考试占总成绩的50%,平时作业占总成绩的30%,课堂表现占总成绩的20%。
2. 按照设定的权重计算每个考核部分的得分,比如期末考试得了80分,平时作业得了90分,课堂表现得了85分。
3. 将每个考核部分得分乘以对应的权重,然后将它们相加,即可得到总成绩。在这个例子中,总成绩为80*0.5 + 90*0.3 + 85*0.2 = 81.5分。
相关问题
python计算学生总成绩
假设你有一个包含学生姓名、语文成绩、数学成绩、英语成绩的字典列表,可以使用以下代码计算每个学生的总成绩:
```python
students = [
{"name": "Alice", "chinese": 90, "math": 85, "english": 95},
{"name": "Bob", "chinese": 80, "math": 90, "english": 85},
{"name": "Charlie", "chinese": 95, "math": 95, "english": 90},
]
for student in students:
total = student["chinese"] + student["math"] + student["english"]
print(f"{student['name']}'s total score is {total}")
```
输出结果为:
```
Alice's total score is 270
Bob's total score is 255
Charlie's total score is 280
```
你也可以将每个学生的总成绩保存在原始字典中,例如:
```python
for student in students:
student["total"] = student["chinese"] + student["math"] + student["english"]
print(f"{student['name']}'s total score is {student['total']}")
```
这样每个学生的字典会变成:
```python
{"name": "Alice", "chinese": 90, "math": 85, "english": 95, "total": 270}
```
用jupyter写Python计算学生总成绩
好的,我可以为您提供一些代码示例,来计算学生的总成绩。首先,我们需要定义一些学生的成绩数据,可以使用列表和字典来表示:
```python
# 定义学生成绩数据
scores = [
{"name": "张三", "math": 80, "english": 90, "chinese": 70},
{"name": "李四", "math": 75, "english": 85, "chinese": 80},
{"name": "王五", "math": 90, "english": 80, "chinese": 85},
{"name": "赵六", "math": 85, "english": 70, "chinese": 90},
]
```
上面的代码定义了四个学生的成绩数据,包括姓名、数学成绩、英语成绩和语文成绩。接下来,我们可以使用循环语句来遍历每个学生的成绩,并计算他们的总成绩:
```python
# 计算学生总成绩
for student in scores:
total_score = student["math"] + student["english"] + student["chinese"]
print(f"{student['name']} 的总成绩是:{total_score}")
```
上面的代码中,我们使用了 for 循环语句来遍历每个学生的成绩,然后计算他们的总成绩,并使用 print 函数输出结果。运行上面的代码,可以得到以下输出:
```
张三 的总成绩是:240
李四 的总成绩是:240
王五 的总成绩是:255
赵六 的总成绩是:245
```
上面的输出结果显示了每个学生的姓名和总成绩。如果您需要将学生的成绩保存到文件或数据库中,可以使用相应的 Python 库来实现。
相关推荐
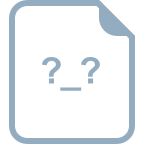
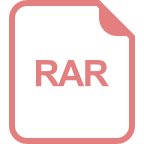












