.创建表t_student,其中包含学号stuno,姓名stuname,性别stusex三个字段,在表中插入一些测试数据。(5分) 2.实现下述功能: 编写显示所有学生资料信息页面,其中包含一个表单用来根据学生姓名模糊查询匹配的学生信息。(50分) 在学生信息后面增加一个“删除学生信息”链接,单击链接可以将学生信息从数据库中删除,然后跳转到显示所有学生资料信息页面。(25分)尝试实现学生信息的添加和修改功能。
时间: 2023-09-17 10:11:53 浏览: 252
1. 创建表t_student并插入测试数据:
```
CREATE TABLE t_student (
stuno VARCHAR(10) PRIMARY KEY,
stuname VARCHAR(20) NOT NULL,
stusex CHAR(2) CHECK(stusex IN ('男', '女'))
);
INSERT INTO t_student (stuno, stuname, stusex)
VALUES ('1001', '张三', '男'), ('1002', '李四', '女'), ('1003', '王五', '男');
```
2. 实现显示所有学生资料信息页面:
```
<!DOCTYPE html>
<html>
<head>
<title>学生信息管理系统</title>
</head>
<body>
<h1>学生信息列表</h1>
<form action="" method="get">
<label>姓名:</label>
<input type="text" name="keyword" value="<?php echo isset($_GET['keyword']) ? $_GET['keyword'] : ''; ?>">
<input type="submit" value="查询">
</form>
<table border="1">
<tr>
<th>学号</th>
<th>姓名</th>
<th>性别</th>
<th>操作</th>
</tr>
<?php
// 连接数据库
$dsn = 'mysql:host=localhost;dbname=test';
$username = 'root';
$password = '123456';
$conn = new PDO($dsn, $username, $password);
// 查询学生信息
$sql = 'SELECT * FROM t_student';
if (isset($_GET['keyword']) && !empty($_GET['keyword'])) {
$keyword = $_GET['keyword'];
$sql .= " WHERE stuname LIKE '%$keyword%'";
}
$stmt = $conn->prepare($sql);
$stmt->execute();
$result = $stmt->fetchAll(PDO::FETCH_ASSOC);
// 显示学生信息
foreach ($result as $row) {
echo '<tr>';
echo '<td>' . $row['stuno'] . '</td>';
echo '<td>' . $row['stuname'] . '</td>';
echo '<td>' . $row['stusex'] . '</td>';
echo '<td><a href="delete.php?stuno=' . $row['stuno'] . '">删除</a></td>';
echo '</tr>';
}
// 关闭数据库连接
$conn = null;
?>
</table>
</body>
</html>
```
3. 实现删除学生信息功能:
```
<?php
// 获取学号
$stuno = $_GET['stuno'];
// 连接数据库
$dsn = 'mysql:host=localhost;dbname=test';
$username = 'root';
$password = '123456';
$conn = new PDO($dsn, $username, $password);
// 删除学生信息
$sql = 'DELETE FROM t_student WHERE stuno = ?';
$stmt = $conn->prepare($sql);
$stmt->bindParam(1, $stuno);
$stmt->execute();
// 关闭数据库连接
$conn = null;
// 跳转到显示所有学生资料信息页面
header('Location: index.php');
exit;
?>
```
4. 实现添加学生信息功能:
```
<!DOCTYPE html>
<html>
<head>
<title>添加学生信息</title>
</head>
<body>
<h1>添加学生信息</h1>
<form action="save.php" method="post">
<label>学号:</label>
<input type="text" name="stuno" required>
<br>
<label>姓名:</label>
<input type="text" name="stuname" required>
<br>
<label>性别:</label>
<input type="radio" name="stusex" value="男" required>男
<input type="radio" name="stusex" value="女" required>女
<br>
<input type="submit" value="添加">
</form>
</body>
</html>
```
```
<?php
// 获取表单数据
$stuno = $_POST['stuno'];
$stuname = $_POST['stuname'];
$stusex = $_POST['stusex'];
// 连接数据库
$dsn = 'mysql:host=localhost;dbname=test';
$username = 'root';
$password = '123456';
$conn = new PDO($dsn, $username, $password);
// 插入学生信息
$sql = 'INSERT INTO t_student (stuno, stuname, stusex) VALUES (?, ?, ?)';
$stmt = $conn->prepare($sql);
$stmt->bindParam(1, $stuno);
$stmt->bindParam(2, $stuname);
$stmt->bindParam(3, $stusex);
$stmt->execute();
// 关闭数据库连接
$conn = null;
// 跳转到显示所有学生资料信息页面
header('Location: index.php');
exit;
?>
```
5. 实现修改学生信息功能:
```
<!DOCTYPE html>
<html>
<head>
<title>修改学生信息</title>
</head>
<body>
<h1>修改学生信息</h1>
<?php
// 获取学号
$stuno = $_GET['stuno'];
// 连接数据库
$dsn = 'mysql:host=localhost;dbname=test';
$username = 'root';
$password = '123456';
$conn = new PDO($dsn, $username, $password);
// 查询学生信息
$sql = 'SELECT * FROM t_student WHERE stuno = ?';
$stmt = $conn->prepare($sql);
$stmt->bindParam(1, $stuno);
$stmt->execute();
$result = $stmt->fetch(PDO::FETCH_ASSOC);
// 显示学生信息表单
echo '<form action="update.php" method="post">';
echo '<input type="hidden" name="stuno" value="' . $result['stuno'] . '">';
echo '<label>姓名:</label>';
echo '<input type="text" name="stuname" value="' . $result['stuname'] . '" required>';
echo '<br>';
echo '<label>性别:</label>';
echo '<input type="radio" name="stusex" value="男" ' . ($result['stusex'] == '男' ? 'checked' : '') . ' required>男';
echo '<input type="radio" name="stusex" value="女" ' . ($result['stusex'] == '女' ? 'checked' : '') . ' required>女';
echo '<br>';
echo '<input type="submit" value="保存">';
echo '</form>';
// 关闭数据库连接
$conn = null;
?>
</body>
</html>
```
```
<?php
// 获取表单数据
$stuno = $_POST['stuno'];
$stuname = $_POST['stuname'];
$stusex = $_POST['stusex'];
// 连接数据库
$dsn = 'mysql:host=localhost;dbname=test';
$username = 'root';
$password = '123456';
$conn = new PDO($dsn, $username, $password);
// 更新学生信息
$sql = 'UPDATE t_student SET stuname = ?, stusex = ? WHERE stuno = ?';
$stmt = $conn->prepare($sql);
$stmt->bindParam(1, $stuname);
$stmt->bindParam(2, $stusex);
$stmt->bindParam(3, $stuno);
$stmt->execute();
// 关闭数据库连接
$conn = null;
// 跳转到显示所有学生资料信息页面
header('Location: index.php');
exit;
?>
```
阅读全文
相关推荐
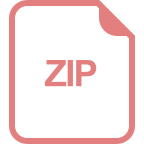
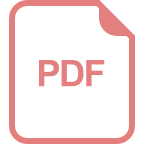
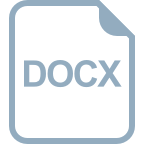














