python获取excel文件
时间: 2023-11-29 19:41:37 浏览: 29
要使用Python获取Excel文件,可以按照以下步骤进行操作:
1. 导入xlrd库:使用pip install xlrd命令安装xlrd库,然后在代码中导入该库。
2. 读取Excel文件:使用xlrd.open_workbook()函数打开Excel文件,并将返回的对象保存到一个变量中。
3. 选择所需的sheet:使用sheet_by_name()函数或sheet_by_index()函数选择要操作的sheet,并将返回的对象保存到一个变量中。
4. 获取行列数:使用nrows属性获取sheet的行数,使用ncols属性获取sheet的列数,并将结果打印出来。
下面是一个示例代码:
```python
import xlrd
# 读取Excel文件
readfile = xlrd.open_workbook(r"E:\PycharmProjects\APITest\工作簿1.xls")
# 选择所需的sheet
obj_sheet = readfile.sheet_by_name("name")
# 获取行列数
row = obj_sheet.nrows
col = obj_sheet.ncols
print("行数:", row)
print("列数:", col)
```
相关问题
python读取excel文件
### 回答1:
可以使用 pandas 库中的 read_excel() 函数来读取 Excel 文件,示例代码如下:
```python
import pandas as pd
# 读取 Excel 文件
df = pd.read_excel('example.xlsx', sheet_name='Sheet1')
# 打印读取的数据
print(df)
```
其中,'example.xlsx' 是 Excel 文件的路径,'Sheet1' 是要读取的工作表名称。read_excel() 函数还有很多参数可以设置,具体可以参考 pandas 文档。
### 回答2:
Python读取Excel文件可以使用第三方库如`pandas`和`openpyxl`。下面以`pandas`为例介绍如何读取Excel文件。
1. 安装pandas库:在命令行中运行`pip install pandas`命令,安装最新版本的pandas库。
2. 导入pandas库:在Python代码中导入pandas库,`import pandas as pd`。
3. 使用pandas读取Excel文件:使用`pd.read_excel()`函数来读取Excel文件。例如,如果要读取名为`data.xlsx`的Excel文件,可以使用以下代码:
```python
data = pd.read_excel('data.xlsx')
```
这将把Excel文件中的数据读取到一个名为`data`的DataFrame对象中。
4. 处理Excel数据:读取Excel文件后,可以对数据进行各种操作,如筛选、排序、计算等。例如,可以使用`head()`函数查看前几行数据:
```python
print(data.head())
```
5. 保存结果:如果需要将处理后的数据保存为Excel文件,可以使用`to_excel()`函数。例如,将处理后的数据保存为名为`result.xlsx`的文件:
```python
data.to_excel('result.xlsx', index=False)
```
这将生成一个不包含索引的Excel文件。
总结:使用`pandas`库的`read_excel()`函数可以方便地读取Excel文件,然后可以对数据进行各种处理和保存。通过这些简单的步骤,我们可以轻松地在Python中读取和处理Excel文件。
### 回答3:
Python读取Excel文件的主要方式是使用第三方库:openpyxl和pandas。
使用openpyxl库可以实现对Excel文件的读取和写入操作。首先,我们需要安装openpyxl库。然后,导入openpyxl模块,使用`load_workbook`函数加载Excel文件,再选择指定的工作表。接着,我们可以通过读取单元格的值、行或列的数据等方式来获取Excel文件中的数据。
另一个常用的库是pandas,它提供了更高级的Excel文件读取和处理功能。首先,我们需要安装pandas库。然后,导入pandas模块,使用`read_excel`函数来读取Excel文件。这个函数可以直接读取整个Excel文件或选择指定的工作表。我们可以通过DataFrame数据结构或`values`属性来获取Excel文件中的数据。
无论使用openpyxl还是pandas,读取Excel文件的过程中,我们需要注意文件路径的正确设置,并确保Excel文件格式正确。
总的来说,Python读取Excel文件的过程需要依托openpyxl或pandas等库来实现。通过这些库,我们可以方便地读取Excel文件中的数据,并进行后续的处理和分析。
python读取EXCEL文件
要使用Python读取Excel文件,首先需要安装xlrd库。可以使用以下命令安装xlrd库:pip install xlrd。然后,可以使用xlrd的open_workbook函数打开Excel文件,指定文件路径作为参数。接下来,可以使用xlrd提供的方法来读取Excel文件中的数据,例如,使用cell_value方法获取单元格数据,使用row_values方法获取行数据,使用col_values方法获取列数据。以下是一个示例代码:
```python
import xlrd
# 打开Excel文件
readfile = xlrd.open_workbook(r"E:\PycharmProjects\APITest\工作簿1.xls")
# 获取所有的Sheet名称
sheet_names = readfile.sheet_names()
print("Sheet名称:", sheet_names)
# 选择第一个Sheet
obj_sheet = readfile.sheet_by_index(0)
# 获取行数和列数
row = obj_sheet.nrows
col = obj_sheet.ncols
# 获取单元格数据
for i in range(row):
for j in range(col):
print(obj_sheet.cell_value(i, j))
# 获取行数据
for i in range(row):
print(obj_sheet.row_values(i))
# 获取列数据
for i in range(col):
print(obj_sheet.col_values(i))
```
相关推荐
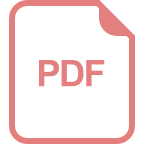




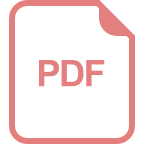
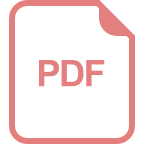
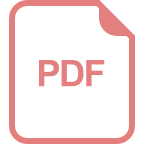
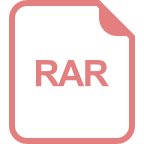
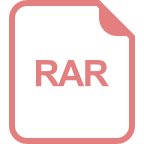
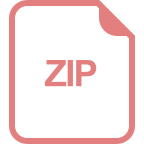