How to implementation queueby the follow struct? struct QueueRecord{ int Front; int Rear; ElementType Array[MaxSize]; };
时间: 2024-10-25 11:16:24 浏览: 29
To implement a queue using the given structure `QueueRecord`, you need to maintain two pointers: `Front` and `Rear`. The `Front` points to the front element of the queue, and the `Rear` points to the next available position where an element can be added. The `Array` serves as the storage for the elements.
Here's a step-by-step explanation of how to implement basic operations like enqueue (enqueue an element), dequeue (remove and return the front element), and checking if the queue is empty or full:
1. **Initialization**:
Create an instance of `QueueRecord` with both `Front` and `Rear` set to `-1` (or 0 in some implementations, representing an empty queue):
```c
QueueRecord queue;
queue.Front = -1;
queue.Rear = -1;
```
2. **Enqueue (enqueue an element)**:
- Check if the queue is full (i.e., `(Rear + 1) % MaxSize == Front`). If not full, increment `Rear` and add the element at `Array[Rear]`.
```c
void enqueue(QueueRecord *queue, ElementType item) {
if ((queue->Rear + 1) % MaxSize != queue->Front) {
queue->Array[queue->Rear] = item;
queue->Rear = (queue->Rear + 1) % MaxSize;
} else {
printf("Queue is full.\n");
}
}
```
3. **Dequeue (remove and return the front element)**:
- Check if the queue is empty (i.e., `queue->Front == queue->Rear`). If not empty, store the front element in a temporary variable, increment `Front`, and return it.
```c
ElementType dequeue(QueueRecord *queue) {
if (queue->Front == queue->Rear) {
printf("Queue is empty.\n");
return NULL; // Return NULL or throw an exception
}
ElementType frontItem = queue->Array[queue->Front];
queue->Front = (queue->Front + 1) % MaxSize;
return frontItem;
}
```
4. **Checking queue status**:
- Use `is_empty()` and `is_full()` functions to check if the queue is empty or full, respectively, based on the current values of `Front` and `Rear`.
Remember that the `%` operator is used here to ensure circular buffer behavior, wrapping around when `Rear` reaches the end (`MaxSize`).
阅读全文
相关推荐













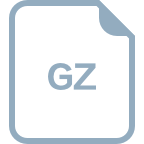

