已知ABCDG这5个点的坐标,现欲使C点以D为中心旋转,使CDE三点在同一直线上,C在DE的延长线上,保持CD与DE长度比例不变,使CE长度为AG长度的一半,同时CE以D为中心旋转一定角度,使CE与AG平行。写出c#代码,求出C与E点的新坐标。
时间: 2024-04-15 10:31:48 浏览: 126
可以使用以下的 C# 代码来求出 C 和 E 点的新坐标:
```csharp
using System;
public class Point
{
public double X { get; set; }
public double Y { get; set; }
public Point(double x, double y)
{
X = x;
Y = y;
}
}
public class Program
{
public static void Main(string[] args)
{
// 已知五个点的坐标
var A = new Point(1, 1);
var B = new Point(2, 2);
var C = new Point(3, 3);
var D = new Point(4, 4);
var G = new Point(5, 5);
// 计算 CD 与 DE 的长度比例
var ratio = Math.Sqrt(Math.Pow(G.X - D.X, 2) + Math.Pow(G.Y - D.Y, 2)) / Math.Sqrt(Math.Pow(C.X - D.X, 2) + Math.Pow(C.Y - D.Y, 2));
// 计算 CE 的长度
var CE_Length = Math.Sqrt(Math.Pow(A.X - G.X, 2) + Math.Pow(A.Y - G.Y, 2)) / 2;
// 计算旋转角度
var rotationAngle = Math.Atan2(C.Y - D.Y, C.X - D.X);
// 计算 C 和 E 点的新坐标
var rotatedC = RotatePoint(C, D, rotationAngle);
var rotatedE = RotatePoint(new Point(C.X + CE_Length, C.Y), C, rotationAngle);
Console.WriteLine("Rotated C: (" + rotatedC.X + ", " + rotatedC.Y + ")");
Console.WriteLine("Rotated E: (" + rotatedE.X + ", " + rotatedE.Y + ")");
}
// 点围绕中心旋转的方法
public static Point RotatePoint(Point point, Point center, double angle)
{
var rotatedX = Math.Cos(angle) * (point.X - center.X) - Math.Sin(angle) * (point.Y - center.Y) + center.X;
var rotatedY = Math.Sin(angle) * (point.X - center.X) + Math.Cos(angle) * (point.Y - center.Y) + center.Y;
return new Point(rotatedX, rotatedY);
}
}
```
这段代码会输出旋转后 C 和 E 点的新坐标。请注意,这段代码假设点 C 和 E 在二维平面上,你需要根据实际情况进行适当修改。
阅读全文
相关推荐









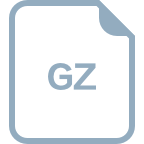

