用c语言二叉排序树非递归插入
时间: 2024-09-21 21:12:04 浏览: 57
在 C 语言中,非递归插入节点到二叉排序树(BST,Binary Search Tree)通常是通过迭代的方式来实现的,因为递归可能会导致栈溢出,特别是当树深度很大时。以下是基本步骤:
1. 定义一个指向当前访问位置的指针,通常初始化为根节点。
2. 比较待插入值与当前节点值的大小关系:
- 如果新值小于当前节点,向左子树移动,更新当前节点为左子节点。
- 如果新值大于当前节点,向右子树移动,更新当前节点为右子节点。
3. 当找到空的位置(null节点)时,将新值插入到该位置。
4. 使用循环遍历直到找到合适的位置,而不是使用递归函数。
下面是一个简单的示例代码片段,假设我们有一个 `Node` 结构体表示BST的节点:
```c
typedef struct Node {
int data;
struct Node* left;
struct Node* right;
} Node;
void insert(Node** root, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (*root == NULL) {
*root = newNode;
} else {
Node* currentNode = *root;
while (true) {
if (value < currentNode->data) {
if (currentNode->left == NULL) {
currentNode->left = newNode;
break;
}
currentNode = currentNode->left;
} else {
if (currentNode->right == NULL) {
currentNode->right = newNode;
break;
}
currentNode = currentNode->right;
}
}
}
}
```
阅读全文
相关推荐
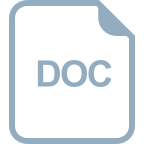
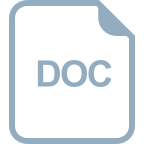
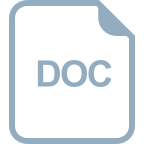


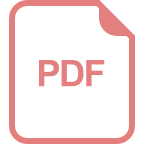
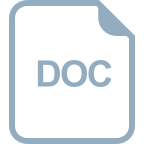


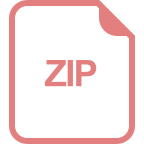
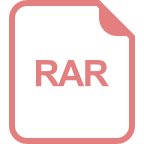
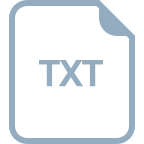
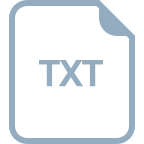
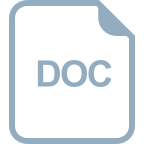

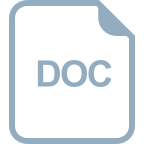