c语言计算二叉排序树深度
时间: 2023-11-28 19:43:51 浏览: 120
以下是C语言计算二叉排序树深度的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 二叉树结构体
typedef struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
// 创建二叉树
TreeNode* createTree(int val) {
TreeNode* node = (TreeNode*)malloc(sizeof(TreeNode));
node->val = val; node->left = NULL;
node->right = NULL;
return node;
}
// 插入节点
void insertNode(TreeNode* root, int val) {
if (root == NULL) {
return;
}
if (val < root->val) {
if (root->left == NULL) {
root->left = createTree(val);
} else {
insertNode(root->left, val);
}
} else {
if (root->right == NULL) {
root->right = createTree(val);
} else {
insertNode(root->right, val);
}
}
}
// 计算二叉树深度
int getDepth(TreeNode* root) {
if (root == NULL) {
return 0;
}
int leftDepth = getDepth(root->left);
int rightDepth = getDepth(root->right);
return (leftDepth > rightDepth ? leftDepth : rightDepth) + 1;
}
int main() {
// 创建二叉排序树
TreeNode* root = createTree(5);
insertNode(root, 3);
insertNode(root, 7);
insertNode(root, 1);
insertNode(root, 9);
insertNode(root, 4);
insertNode(root, 6);
// 计算二叉排序树深度
int depth = getDepth(root);
printf("The depth of the binary sort tree is: %d\n", depth);
return 0;
}
```
阅读全文
相关推荐

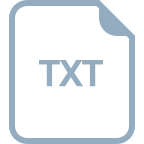
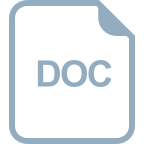
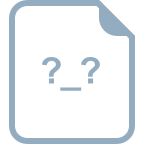







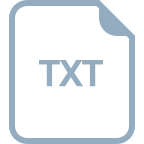
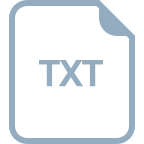
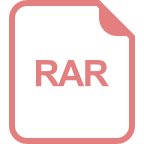
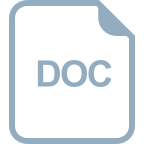