写一个求二叉排序树深度的c语言代码
时间: 2023-11-04 15:27:19 浏览: 83
以下是求二叉排序树深度的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct TreeNode {
int value;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
int maxDepth(TreeNode *root) {
if (root == NULL) {
return 0;
}
int leftDepth = maxDepth(root->left);
int rightDepth = maxDepth(root->right);
return leftDepth > rightDepth ? leftDepth + 1 : rightDepth + 1;
}
int main() {
TreeNode *root = (TreeNode*) malloc(sizeof(TreeNode));
root->value = 4;
root->left = (TreeNode*) malloc(sizeof(TreeNode));
root->left->value = 2;
root->left->left = (TreeNode*) malloc(sizeof(TreeNode));
root->left->left->value = 1;
root->left->left->left = NULL;
root->left->left->right = NULL;
root->left->right = (TreeNode*) malloc(sizeof(TreeNode));
root->left->right->value = 3;
root->left->right->left = NULL;
root->left->right->right = NULL;
root->right = (TreeNode*) malloc(sizeof(TreeNode));
root->right->value = 6;
root->right->left = (TreeNode*) malloc(sizeof(TreeNode));
root->right->left->value = 5;
root->right->left->left = NULL;
root->right->left->right = NULL;
root->right->right = (TreeNode*) malloc(sizeof(TreeNode));
root->right->right->value = 7;
root->right->right->left = NULL;
root->right->right->right = NULL;
int depth = maxDepth(root);
printf("The depth of the binary search tree is %d.\n", depth);
return 0;
}
```
在这个代码示例中,我们首先定义了一个 `TreeNode` 结构体表示二叉排序树中的节点,然后实现了一个 `maxDepth` 函数来计算二叉排序树的深度。该函数使用递归的方式遍历整棵树,返回左子树和右子树的深度的最大值再加上1,即为整棵树的深度。最后,我们在主函数中构造了一个测试用的二叉排序树,并调用 `maxDepth` 函数计算其深度并输出结果。
阅读全文
相关推荐



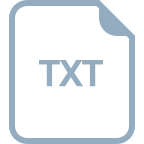
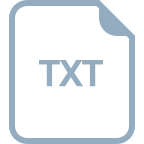
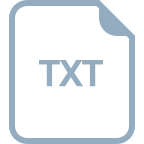
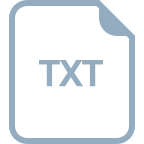
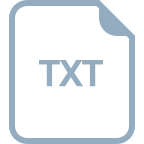
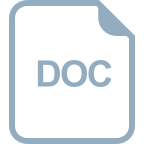
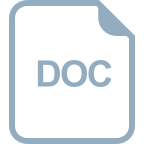
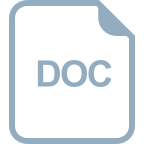




