Python 用GeoPandas库实现加载shp文件并绘制地图显示数据,可以新增删除点、线、面
时间: 2024-06-11 13:10:15 浏览: 9
首先需要安装GeoPandas库,可以使用以下命令安装:
```
pip install geopandas
```
接下来,我们可以使用以下代码加载一个shp文件:
```
import geopandas as gpd
# 加载shp文件
shapefile = gpd.read_file("path/to/shapefile.shp")
# 显示数据
print(shapefile.head())
```
此时,我们可以看到shp文件的前几行数据。
接下来,我们可以使用以下代码绘制地图并将数据显示在地图上:
```
import matplotlib.pyplot as plt
# 绘制地图
shapefile.plot()
# 显示地图
plt.show()
```
此时,我们可以看到一个简单的地图显示了出来。
如果想要新增点、线或面,可以使用以下代码:
```
from shapely.geometry import Point, LineString, Polygon
# 新增点
point = Point(x, y)
shapefile = shapefile.append({'geometry': point}, ignore_index=True)
# 新增线
line = LineString([(x1, y1), (x2, y2)])
shapefile = shapefile.append({'geometry': line}, ignore_index=True)
# 新增面
polygon = Polygon([(x1, y1), (x2, y2), (x3, y3)])
shapefile = shapefile.append({'geometry': polygon}, ignore_index=True)
```
其中,x、y、x1、y1、x2、y2、x3、y3为点的坐标。
如果想要删除点、线或面,可以使用以下代码:
```
# 删除第n行数据
shapefile = shapefile.drop(n)
```
其中,n为需要删除的行数。
综上所述,使用GeoPandas库可以轻松加载shp文件并绘制地图,同时也可以实现新增、删除点、线、面等操作。
相关推荐
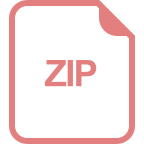














