cuda中如何使用eigen的矩阵乘法
时间: 2024-03-27 08:36:36 浏览: 72
在CUDA中使用Eigen进行矩阵乘法,需要使用Eigen Cuda库,具体使用步骤如下:
1. 引入Eigen和Eigen Cuda的头文件
```
#include <Eigen/Dense>
#include <Eigen/CudaDense>
```
2. 创建GPU设备
```
CudaDevice device;
```
3. 在GPU设备上分配内存并将数据拷贝到GPU
```
MatrixXd mat1 = MatrixXd::Random(1000, 1000);
MatrixXd mat2 = MatrixXd::Random(1000, 1000);
MatrixXd mat3 = device.allocate(1000, 1000);
mat3 = mat1 * mat2;
```
在上述代码中,首先使用MatrixXd创建CPU上的矩阵mat1和mat2,然后使用CudaDevice的allocate函数在GPU上分配内存,并将结果保存在mat3中。
4. 将结果从GPU拷贝回CPU
```
MatrixXd result = mat3;
```
5. 完整代码示例
```
#include <iostream>
#include <Eigen/Dense>
#include <Eigen/CudaDense>
using namespace Eigen;
using namespace Eigen::cuda;
using namespace std;
int main()
{
CudaDevice device;
MatrixXd mat1 = MatrixXd::Random(1000, 1000);
MatrixXd mat2 = MatrixXd::Random(1000, 1000);
MatrixXd mat3 = device.allocate(1000, 1000);
mat3 = mat1 * mat2;
MatrixXd result = mat3;
cout << result << endl;
return 0;
}
```
以上是在CUDA中使用Eigen进行矩阵乘法的基本步骤,需要注意的是,在GPU上进行矩阵运算需要消耗大量的内存和计算资源,建议在进行大规模矩阵运算前进行性能测试。
阅读全文
相关推荐
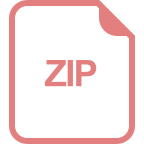
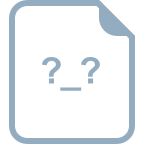
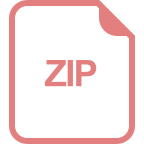
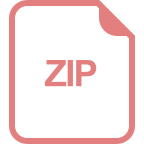
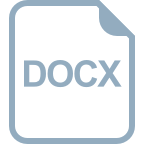
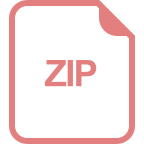
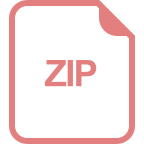
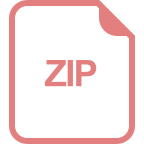
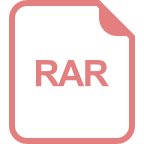
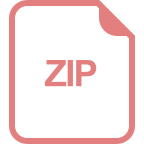