python的circle(rad)
时间: 2024-09-12 21:14:59 浏览: 78
Python中的circle(rad)不是一个内置函数或方法。但是,如果你想要创建一个表示圆的对象,并且使用半径(rad)作为参数,你可以定义一个类来实现这个功能。下面是一个简单的例子:
```python
import math
class Circle:
def __init__(self, radius):
self.radius = radius
def circumference(self):
return 2 * math.pi * self.radius
def area(self):
return math.pi * self.radius ** 2
```
在这个例子中,我们定义了一个Circle类,它接受一个半径参数来初始化一个圆。然后,我们定义了两个方法:`circumference`用于计算圆的周长,`area`用于计算圆的面积。这里使用了`math.pi`来获取圆周率π的值。
使用这个类创建圆的对象,并调用相关方法的例子如下:
```python
# 创建一个半径为5的圆
my_circle = Circle(5)
# 计算并打印圆的周长和面积
print("圆的周长:", my_circle.circumference())
print("圆的面积:", my_circle.area())
```
这段代码会输出圆的周长和面积,基于半径为5的参数。
相关问题
opencv python 曲线拟合
以下是两种使用OpenCV进行曲线拟合的例子:
1. 最小外包圆形拟合:
```python
import cv2 as cv
import numpy as np
image = cv.imread("huzi.jpeg")
cv.imshow("image", image)
gray = cv.cvtColor(image, cv.COLOR_BGR2GRAY)
ret, binary = cv.threshold(gray, 127, 255, cv.THRESH_BINARY)
contours, hierarchy = cv.findContours(binary, cv.RETR_LIST, cv.CHAIN_APPROX_SIMPLE)
(x, y), rad = cv.minEnclosingCircle(contours[0])
center = (int(x), int(y))
rad = int(rad)
cv.circle(image, center, rad, (255, 255, 255), 1)
cv.imshow("result", image)
cv.waitKey()
cv.destroyAllWindows()
```
2. 最小外包椭圆拟合:
```python
import cv2 as cv
import numpy as np
image = cv.imread("huzi.jpeg")
cv.imshow("image", image)
gray = cv.cvtColor(image, cv.COLOR_BGR2GRAY)
ret, binary = cv.threshold(gray, 127, 255, cv.THRESH_BINARY)
contours, hierarchy = cv.findContours(binary, cv.RETR_LIST, cv.CHAIN_APPROX_SIMPLE)
ellipse = cv.fitEllipse(contours[0])
cv.ellipse(image, ellipse, (255, 255, 0), 1)
cv.imshow("result", image)
cv.waitKey()
cv.destroyAllWindows()
```
python太极旋转动画
Python太极旋转动画是一种使用Python编程语言和图形库(如Matplotlib或Pygame)来创建太极图并进行旋转动画的效果。以下是一个使用Matplotlib库创建太极旋转动画的示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
# 创建图形和坐标轴
fig, ax = plt.subplots()
ax.set_aspect('equal')
ax.axis('off')
# 绘制太极图
def draw_taiji():
# 绘制大圆
circle = plt.Circle((0, 0), 1, color='black', fill=True)
ax.add_patch(circle)
# 绘制小圆
small_circle1 = plt.Circle((0, 0.5), 0.5, color='white', fill=True)
small_circle2 = plt.Circle((0, -0.5), 0.5, color='black', fill=True)
ax.add_patch(small_circle1)
ax.add_patch(small_circle2)
# 绘制小圆中的小圆
small_circle3 = plt.Circle((0, 0.5), 0.1, color='black', fill=True)
small_circle4 = plt.Circle((0, -0.5), 0.1, color='white', fill=True)
ax.add_patch(small_circle3)
ax.add_patch(small_circle4)
# 初始化动画
def init():
draw_taiji()
return []
# 更新动画帧
def update(frame):
ax.cla()
ax.set_aspect('equal')
ax.axis('off')
# 旋转太极图
angle = np.deg2rad(frame)
rotation_matrix = np.array([[np.cos(angle), -np.sin(angle)],
[np.sin(angle), np.cos(angle)]])
# 绘制太极图
draw_taiji()
# 旋转所有图形元素
for patch in ax.patches:
vertices = patch.get_path().vertices
rotated_vertices = np.dot(vertices, rotation_matrix)
patch.set_xy(rotated_vertices)
return []
# 创建动画
ani = FuncAnimation(fig, update, frames=range(0, 360, 2), init_func=init, blit=True, interval=50)
plt.show()
```
这段代码使用Matplotlib库创建了一个太极旋转动画。`draw_taiji`函数用于绘制太极图,`update`函数用于更新每一帧的动画,`FuncAnimation`类用于创建动画并显示。
阅读全文
相关推荐
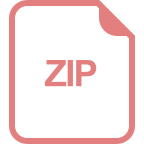
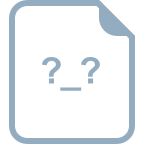
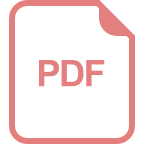
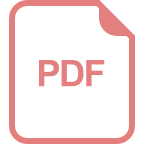
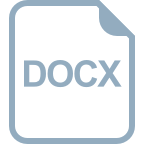
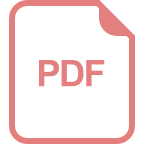
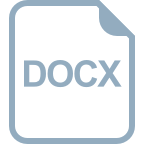
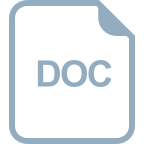
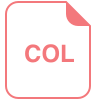





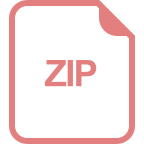
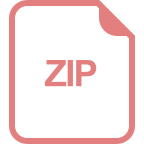