汉诺塔的非递归实现c++
时间: 2023-12-06 12:54:00 浏览: 39
汉诺塔问题的非递归实现需要借助栈来完成。下面是C语言实现的非递归汉诺塔算法:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct _stack {
int *data; // 存储栈中的数据
int top; // 栈顶位置
int capacity; // 栈的容量
} stack;
// 创建一个指定容量的栈
stack *createStack(int capacity) {
stack *s = (stack *)malloc(sizeof(stack));
s->data = (int *)malloc(sizeof(int) * capacity);
s->top = -1;
s->capacity = capacity;
return s;
}
// 判断栈是否为空
int isStackEmpty(stack *s) {
return s->top == -1;
}
// 判断栈是否已满
int isStackFull(stack *s) {
return s->top == s->capacity - 1;
}
// 入栈
void push(stack *s, int value) {
if (isStackFull(s)) {
printf("Stack overflow.\n");
return;
}
s->data[++s->top] = value;
}
// 出栈
int pop(stack *s) {
if (isStackEmpty(s)) {
printf("Stack underflow.\n");
return -1;
}
return s->data[s->top--];
}
// 汉诺塔的非递归实现
void hanoi(int n) {
stack *s = createStack(n);
int i, x, step = 0;
for (i = n; i >= 1; i--) {
push(s, i);
}
while (!isStackEmpty(s)) {
x = pop(s);
step++;
if (isStackEmpty(s)) {
printf("Move disk %d from A to C.\n", x);
continue;
}
push(s, x);
if (step % 2 == 1) {
x = pop(s);
push(s, x);
printf("Move disk %d from A to B.\n", x);
} else {
x = pop(s);
push(s, x);
printf("Move disk %d from B to C.\n", x);
}
}
free(s->data);
free(s);
}
int main() {
int n;
printf("Please input the number of disks: ");
scanf("%d", &n);
hanoi(n);
return 0;
}
```
该算法使用了一个栈来存储盘子,并对盘子进行移动。具体实现过程可以参考代码注释。
相关推荐
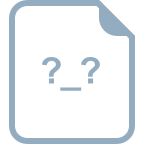
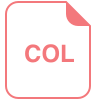













