求出一个带权完全二叉树每一层的权重,然后找出最大的权重。若是有权重相等的层数,就返回权重最小的那一 层。代码C语言
时间: 2024-10-22 09:27:16 浏览: 60
在C语言中,我们可以使用递归的方式来求解这个问题。首先,我们需要定义一个辅助函数来计算单个节点的深度(即从根到该节点的距离),然后遍历整个二叉树。这里是一个简单的示例:
```c
#include <stdio.h>
// 定义一个结构体表示树节点
typedef struct TreeNode {
int weight;
struct TreeNode *left, *right;
} TreeNode;
// 计算节点深度
int getDepth(TreeNode* node) {
if (node == NULL)
return 0;
else
return 1 + max(getDepth(node->left), getDepth(node->right));
}
// 找出最大权重和对应的层数
int findMaxWeightAndLevel(TreeNode* root) {
if (root == NULL)
return -1; // 如果根为空,没有树
int maxWeight = root->weight, maxLevel = getDepth(root);
for (TreeNode* node = root->left; node != NULL; node = node->left->left) { // 遍历左子树
int curWeight = node->weight, curLevel = getDepth(node);
if (curWeight > maxWeight || (curWeight == maxWeight && curLevel < maxLevel)) {
maxWeight = curWeight;
maxLevel = curLevel;
}
}
return maxLevel;
}
int main() {
// 假设你已经有了一个带权的二叉树实例,例如 'root'
int result = findMaxWeightAndLevel(root);
printf("The layer with the maximum weight is %d and its weight is %d.\n", result, maxWeight);
return 0;
}
```
在这个例子中,`findMaxWeightAndLevel` 函数首先检查根节点,然后遍历左子树并更新最大权重和对应层次。如果遇到权重相等的情况,它会选择层次更低的那一层。
请注意,这个代码假设输入的带权完全二叉树已经被适当地初始化,并且 `getDepth` 函数能正确地递归计算每个节点的深度。实际应用时,你需要将树的结构和权重存储在一个合适的结构中,并根据实际情况调整上述代码。
阅读全文
相关推荐
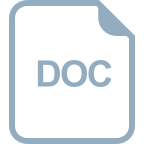
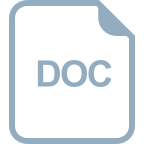
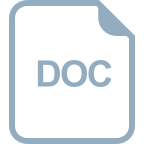

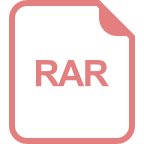
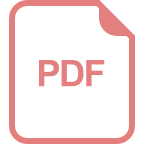
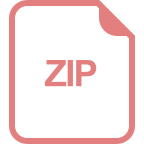
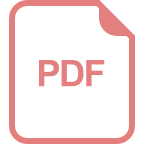
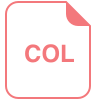

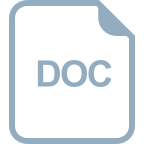
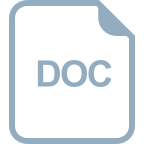
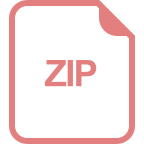
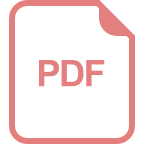
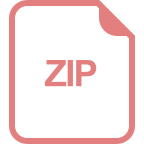
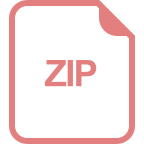
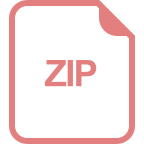
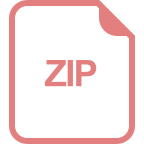