编写一个函数,逆序输出单链表的元素(创建一个链表类,饰演一个方法reverse_print(),使用栈来实现链表的逆序输出)
时间: 2024-09-30 21:09:49 浏览: 40
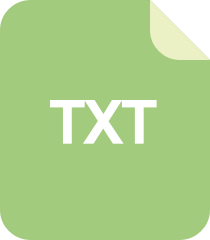
字符串逆序输出
为了编写一个函数,我们将创建一个链表类,并实现一个名为`reverse_print`的方法,利用栈的数据结构来逆序输出链表的元素。下面是一个简单的C++示例:
```cpp
#include <iostream>
#include <stack>
using namespace std;
// 定义链表节点
class ListNode {
public:
int val;
ListNode* next;
ListNode(int x) : val(x), next(nullptr) {}
};
// 链表类
class LinkedList {
private:
ListNode* head;
public:
LinkedList() : head(nullptr) {}
// 添加新元素到链表末尾
void addNode(int val) {
ListNode* newNode = new ListNode(val);
if (!head) {
head = newNode;
} else {
ListNode* current = head;
while (current->next) {
current = current->next;
}
current->next = newNode;
}
}
// 逆序打印链表(使用栈)
void reverse_print() {
stack<int> s;
ListNode* current = head;
// 先将所有元素压入栈中
while (current != nullptr) {
s.push(current->val);
current = current->next;
}
// 从栈顶开始弹出并打印元素
while (!s.empty()) {
cout << s.top() << " ";
s.pop();
}
}
};
int main() {
LinkedList list;
list.addNode(1);
list.addNode(2);
list.addNode(3);
list.addNode(4);
cout << "原始链表: ";
list.reverse_print(); // 输出逆序的链表
return 0;
}
```
在这个例子中,`LinkedList` 类包含了链表的基本操作(添加节点)以及`reverse_print` 函数,该函数通过遍历整个链表并将节点值压入栈中,然后逐个弹出栈顶元素并打印,实现了链表的逆序输出。
阅读全文
相关推荐
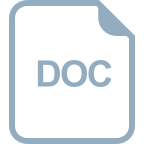
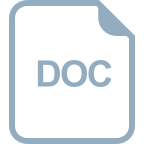

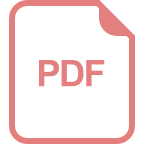
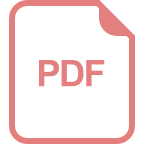
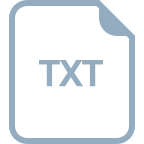
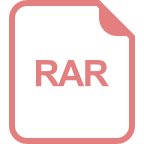
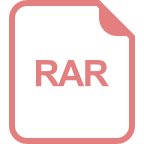
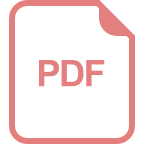
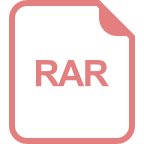
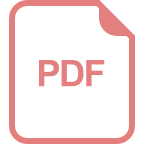
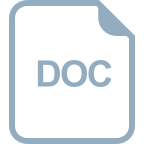
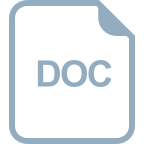
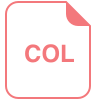


