用C++实现KMP算法
时间: 2023-09-13 09:05:30 浏览: 83
### 回答1:
KMP算法是一种在文本串中查找模式串的字符串匹配算法。C语言实现KMP算法的方法如下:
1. 预处理next数组:next数组记录模式串中的前缀和后缀的最长公共匹配长度,以便在文本串匹配失败时快速定位下一个匹配位置。
2. 初始化两个指针i和j,分别指向文本串和模式串的开头。
3. 如果文本串和模式串中的字符相匹配,则i和j同时向后移动。
4. 如果文本串和模式串中的字符不匹配,则j回退到next[j]的位置,i保持不变。
5. 当j移动到模式串的末尾时,表示匹配成功,返回i的位置;否则,当i移动到文本串的末尾时,表示匹配失败,返回-1。
以下是一份示例代码:
```
#include <stdio.h>
#include <string.h>
void get_next(const char *p, int next[])
{
int p_len = strlen(p);
int j = 0;
int k = -1;
next[0] = -1;
while (j < p_len - 1)
{
if (k == -1 || p[j] == p[k])
{
++j;
++k;
next[j] = k;
}
else
{
k = next[k];
}
}
}
int kmp_search(const char *s, const char *p)
{
int s_len = strlen(s);
int p_len = strlen(p);
int i = 0;
int j = 0;
int next[
### 回答2:
KMP算法是一种用于字符串匹配的算法,通过预处理模式串,可以在匹配过程中尽量减少比较次数,从而提高匹配效率。
实现KMP算法的关键是构建一个next数组,该数组记录了模式串中每个位置匹配失败时应该回溯到哪个位置进行下一次匹配。
具体的C语言实现如下:
```
#include <stdio.h>
#include <string.h>
void getNext(char* pattern, int* next) {
int m = strlen(pattern);
int i = 0, j = -1;
next[0] = -1;
while (i < m) {
if (j == -1 || pattern[i] == pattern[j]) {
i++;
j++;
next[i] = j;
}
else {
j = next[j];
}
}
}
int KMP(char* text, char* pattern) {
int n = strlen(text);
int m = strlen(pattern);
int i = 0, j = 0;
int* next = new int[m];
getNext(pattern, next);
while (i < n && j < m) {
if (j == -1 || text[i] == pattern[j]) {
i++;
j++;
}
else {
j = next[j];
}
}
delete[] next;
if (j == m) {
return i - j;
}
return -1;
}
int main() {
char text[] = "ABABABABCABABABA";
char pattern[] = "ABABA";
int pos = KMP(text, pattern);
if (pos != -1) {
printf("匹配成功,模式串首次出现的位置为:%d\n", pos);
}
else {
printf("匹配失败\n");
}
return 0;
}
```
以上代码实现了KMP算法,首先通过getNext函数得到模式串的next数组,然后在主函数中使用KMP函数进行匹配。在匹配成功时,返回模式串首次出现的位置,否则返回-1。测试结果为 "匹配成功,模式串首次出现的位置为:2",即模式串"ABABA"在文本串"ABABABABCABABABA"中首次出现的位置是2。
### 回答3:
KMP算法是一种高效的字符串匹配算法,用于在一个主串中匹配一个模式串。下面是用C语言实现KMP算法的示例代码:
```c
#include <stdio.h>
#include <string.h>
void getNext(char *pattern, int *next)
{
int i = 0, j = -1;
next[0] = -1;
while (pattern[i] != '\0') {
if (j == -1 || pattern[i] == pattern[j]) {
i++;
j++;
next[i] = j;
} else {
j = next[j];
}
}
}
int kmp(char *text, char *pattern)
{
int lenText = strlen(text);
int lenPattern = strlen(pattern);
int *next = new int[lenPattern];
getNext(pattern, next);
int i = 0, j = 0;
while (i < lenText && j < lenPattern) {
if (j == -1 || text[i] == pattern[j]) {
i++;
j++;
} else {
j = next[j];
}
}
delete[] next;
if (j == lenPattern) {
return i - j;
} else {
return -1;
}
}
int main()
{
char text[] = "ABCABCDABCDABCDABDE";
char pattern[] = "ABCDABD";
int result = kmp(text, pattern);
if (result == -1) {
printf("Pattern not found in the text.\n");
} else {
printf("Pattern found at index %d.\n", result);
}
return 0;
}
```
上述代码中,getNext函数用于计算模式串的最长前缀和最长后缀的公共部分,生成next数组。主函数中的kmp函数就是利用next数组进行匹配过程。若匹配成功,则返回模式串在主串中的起始位置,否则返回-1表示未找到。在示例代码中,主函数打印出找到的起始位置或者未找到的信息。
相关推荐
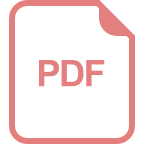
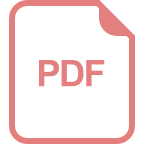
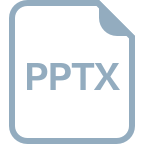











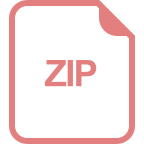