C++ 从函数中形参中返回多个智能指针
时间: 2024-05-10 20:14:30 浏览: 141
在 C++ 中,我们可以使用 `std::tuple` 来从函数中返回多个智能指针。下面是一个示例代码:
```c++
#include <tuple>
#include <memory>
std::tuple<std::unique_ptr<int>, std::shared_ptr<int>> foo() {
// 创建两个智能指针
auto ptr1 = std::make_unique<int>(1);
auto ptr2 = std::make_shared<int>(2);
// 返回一个 tuple
return std::make_tuple(std::move(ptr1), ptr2);
}
int main() {
// 调用 foo() 函数
auto [ptr1, ptr2] = foo();
// 输出智能指针指向的值
std::cout << *ptr1 << ", " << *ptr2 << std::endl;
return 0;
}
```
在这个示例中,`foo()` 函数返回一个 `std::tuple` 对象,其中包含一个 `std::unique_ptr<int>` 和一个 `std::shared_ptr<int>`。在 `main()` 函数中,我们使用结构化绑定来将返回值拆分为两个智能指针,并输出它们指向的值。
相关问题
c++1.用数组作为函数形参实现函数,函数调用时实参为数组。 2.用数组作为函数形参实现函数,函数调用时实参为指针。 3.用指针作为函数形参实现函数,函数调用时实参为数组。 用指针作为函数形参实现函数,函数调用时实参为指针。
1. 用数组作为函数形参实现函数,函数调用时实参为数组:
```c
void func(int arr[], int size) {
// 函数体
}
int main() {
int arr[5] = {1, 2, 3, 4, 5};
func(arr, 5);
return 0;
}
```
2. 用数组作为函数形参实现函数,函数调用时实参为指针:
```c
void func(int *arr, int size) {
// 函数体
}
int main() {
int arr[5] = {1, 2, 3, 4, 5};
func(arr, 5);
return 0;
}
```
3. 用指针作为函数形参实现函数,函数调用时实参为数组:
```c
void func(int *arr, int size) {
// 函数体
}
int main() {
int arr[5] = {1, 2, 3, 4, 5};
func(&arr[0], 5);
return 0;
}
```
4. 用指针作为函数形参实现函数,函数调用时实参为指针:
```c
void func(int *arr, int size) {
// 函数体
}
int main() {
int arr[5] = {1, 2, 3, 4, 5};
func(arr, 5);
return 0;
}
```
注意,指针和数组在函数传参时有一些细微的差别,需要注意。
C++ 函数形参为函数指针,传递bind函数生成的函数对象,编译报错
在C++中,当你尝试将`std::bind`生成的函数对象作为参数传递给另一个函数,尤其是当这个接收函数期望的是函数指针或函数引用时,可能会遇到编译错误。`std::bind`实际上创建了一个可调用对象(Callable Object),它封装了原始函数及其相关的实参。然而,某些函数需要的是直接的函数指针或函数引用,它们无法直接接受这样的可调用对象。
例如:
```cpp
void acceptFunction(int (*func)(int)) { // 接受函数指针
func(42);
}
void passBind(std::function<int(int)> f) { // 接受函数对象
f(42);
}
int add(int a, int b) {
return a + b;
}
int main() {
std::function<int(int)> boundAdd = std::bind(add, 2); // 创建绑定后的函数
// 这行会报错,因为acceptFunction期望函数指针
acceptFunction(boundAdd);
// 这里不会报错,因为passBind可以接受函数对象
passBind(boundAdd);
}
```
在这里,`acceptFunction`调用失败是因为它期望一个函数指针,而`boundAdd`是一个函数对象。解决这个问题的一种方法是适当地修改`acceptFunction`的参数类型,使其能够处理函数对象,或者在调用时使用`.call()`方法来间接地调用函数对象。
如果你遇到了具体的编译错误信息,那通常会提供有关如何修复该问题的线索。常见的错误消息可能包含类似“no matching function for call to 'acceptFunction’”之类的提示。
阅读全文
相关推荐
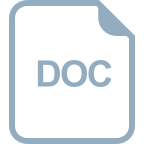
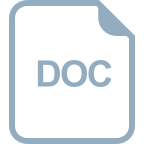
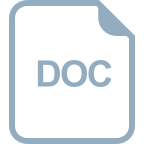
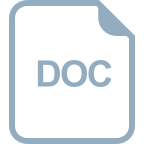








