神经网络中的concate中的参数
时间: 2024-03-04 17:51:27 浏览: 18
在神经网络中,"concate"(即 concatenate)函数通常有两个参数:
1. axis:表示拼接的维度。例如,如果要在 batch 维度上拼接两个张量,那么 axis 应该设置为 0;如果要在 feature 维度上拼接两个张量,那么 axis 应该设置为 -1 或者 3(假设 feature 维度的索引为 3)。
2. tensors:表示要拼接的张量列表。这个参数中的张量应该具有相同的 shape,除了要拼接的维度之外。如果有多个张量需要拼接,那么应该将它们按照拼接的顺序依次传递给这个参数。
相关问题
神经网络中的concate中的参数代码
在 TensorFlow 中,实现 concatenate 函数的代码如下:
```python
import tensorflow as tf
# 假设有两个张量,分别为 tensor1 和 tensor2
# tensor1 的 shape 为 [batch_size, height, width, channel1]
# tensor2 的 shape 为 [batch_size, height, width, channel2]
# 在 feature 维度上拼接 tensor1 和 tensor2
concatenated_tensor = tf.concat([tensor1, tensor2], axis=-1)
# 或者
concatenated_tensor = tf.concat([tensor1, tensor2], axis=3)
```
在 PyTorch 中,实现 concatenate 函数的代码如下:
```python
import torch
# 假设有两个张量,分别为 tensor1 和 tensor2
# tensor1 的 shape 为 [batch_size, channel1, height, width]
# tensor2 的 shape 为 [batch_size, channel2, height, width]
# 在 feature 维度上拼接 tensor1 和 tensor2
concatenated_tensor = torch.cat([tensor1, tensor2], dim=1)
# 或者
concatenated_tensor = torch.cat([tensor1, tensor2], dim=-3)
```
需要注意的是,在 PyTorch 中,dim 参数表示的是拼接的维度的索引,而不是在拼接时的轴名称。因此,如果 feature 维度的索引为 1,那么应该将 dim 设置为 1。
神经网络中实现两个卷积层输出的concate
在神经网络中,将两个卷积层的输出进行拼接(concatenate)通常使用 PyTorch 中的 torch.cat 函数来实现。假设有两个卷积层的输出张量分别为 x1 和 x2,可以使用以下代码将它们在通道维度进行拼接:
``` python
import torch
import torch.nn as nn
# 假设有两个卷积层的输出张量 x1 和 x2
x1 = torch.randn(2, 16, 32, 32) # (batch_size, channels, height, width)
x2 = torch.randn(2, 32, 16, 16) # (batch_size, channels, height, width)
# 定义一个卷积层将两个张量在通道维度拼接
class ConcatConv(nn.Module):
def __init__(self, in_channels, out_channels, kernel_size, stride, padding):
super(ConcatConv, self).__init__()
self.conv = nn.Conv2d(in_channels, out_channels, kernel_size, stride, padding)
def forward(self, x1, x2):
# 在通道维度进行拼接
x = torch.cat([x1, x2], dim=1)
# 卷积操作
x = self.conv(x)
return x
# 创建一个拼接并卷积的层
concat_conv = ConcatConv(48, 64, 3, 1, 1)
# 将 x1 和 x2 输入到拼接层中
y = concat_conv(x1, x2)
print(y.shape) # (2, 64, 32, 32)
```
在上面的代码中,我们定义了一个名为 ConcatConv 的 PyTorch 模块,它包含一个卷积层和一个 forward 方法。在 forward 方法中,我们将 x1 和 x2 在通道维度上进行拼接,并将结果输入到卷积层中进行卷积操作。拼接后的张量的通道数为第一个卷积层输出的通道数和第二个卷积层输出的通道数之和。
需要注意的是,拼接层的输入张量 x1 和 x2 的形状必须相同,除了通道数之外。在上面的代码中,我们假设两个张量的形状分别为 (2, 16, 32, 32) 和 (2, 32, 16, 16),通道维度上的大小分别为 16 和 32。在拼接层中,我们将这两个张量在通道维度上拼接,得到了一个形状为 (2, 48, 32, 32) 的张量,其中通道维度上的大小为 16+32=48。最后,我们将拼接后的张量输入到一个卷积层中进行卷积操作,得到了一个形状为 (2, 64, 32, 32) 的输出张量。
相关推荐
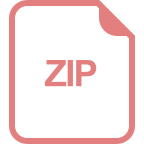







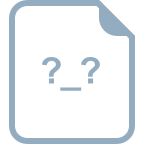
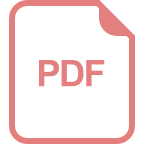
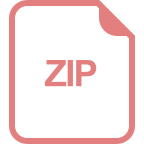
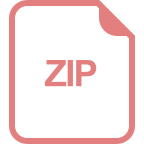
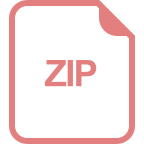
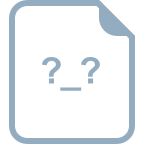