windows下使用C语言调用openssl库生成rsa密钥对,并给出完整代码
时间: 2024-01-24 21:05:25 浏览: 217
好的,下面是一个示例程序,可以在Windows下使用C语言调用openssl库生成RSA密钥对:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/rsa.h>
#include <openssl/pem.h>
int main() {
int ret = 0;
RSA *rsa = NULL;
BIGNUM *e = NULL;
BIO *bio_pub = NULL, *bio_pri = NULL;
char *pub_key = NULL, *pri_key = NULL;
// 生成RSA密钥对
e = BN_new();
ret = BN_set_word(e, RSA_F4);
if (ret != 1) {
printf("BN_set_word failed\n");
return -1;
}
rsa = RSA_new();
ret = RSA_generate_key_ex(rsa, 2048, e, NULL);
if (ret != 1) {
printf("RSA_generate_key_ex failed\n");
return -1;
}
// 生成公钥和私钥
bio_pub = BIO_new(BIO_s_mem());
ret = PEM_write_bio_RSAPublicKey(bio_pub, rsa);
if (ret != 1) {
printf("PEM_write_bio_RSAPublicKey failed\n");
return -1;
}
bio_pri = BIO_new(BIO_s_mem());
ret = PEM_write_bio_RSAPrivateKey(bio_pri, rsa, NULL, NULL, 0, NULL, NULL);
if (ret != 1) {
printf("PEM_write_bio_RSAPrivateKey failed\n");
return -1;
}
// 从BIO中读取公钥和私钥
long pub_len = BIO_get_mem_data(bio_pub, &pub_key);
long pri_len = BIO_get_mem_data(bio_pri, &pri_key);
if (pub_len <= 0 || pri_len <= 0) {
printf("BIO_get_mem_data failed\n");
return -1;
}
// 输出公钥和私钥
printf("public key:\n%s\n", pub_key);
printf("private key:\n%s\n", pri_key);
// 释放资源
RSA_free(rsa);
BN_free(e);
BIO_free_all(bio_pub);
BIO_free_all(bio_pri);
free(pub_key);
free(pri_key);
return 0;
}
```
这个程序使用了openssl库中的RSA函数和PEM函数,生成了一个2048位的RSA密钥对,并且输出了公钥和私钥。需要注意的是,这个程序中的公钥和私钥都是以PEM格式保存的,如果需要使用其他格式,可以使用openssl库中的其他函数进行处理。
阅读全文
相关推荐
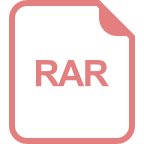
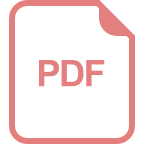
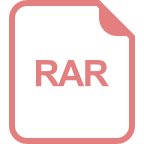
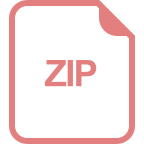
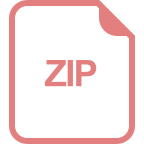
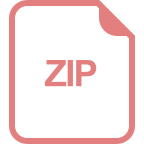
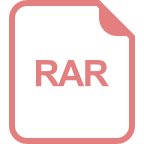
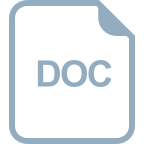
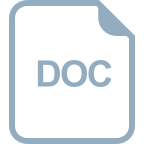
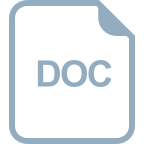




