用VHDL语言,使用两种描述方法,描述半加器电路
时间: 2024-03-15 14:44:09 浏览: 74
方法一:
使用结构化描述法,可以描述出半加器电路的结构和逻辑关系,具体代码如下:
```vhdl
-- 半加器电路结构描述
entity half_adder is
port(a, b : in bit; -- 输入信号 a 和 b
s, c : out bit); -- 输出信号 s 和 c
end half_adder;
architecture structural of half_adder is
begin
s <= a xor b; -- 异或运算得到和
c <= a and b; -- 与运算得到进位
end structural;
```
方法二:
使用行为描述法,可以描述出半加器电路的功能和实现方式,具体代码如下:
```vhdl
-- 半加器电路功能描述
entity half_adder is
port(a, b : in bit; -- 输入信号 a 和 b
s, c : out bit); -- 输出信号 s 和 c
end half_adder;
architecture behavioral of half_adder is
begin
-- 和的计算
s <= a xor b;
-- 进位的计算
c <= a and b;
end behavioral;
```
相关问题
用VHDL语言,使用两种描述方法,描述半加器电路
好的,我明白了。下面我将使用行为级和结构级两种描述方法来描述半加器电路。
首先是行为级描述方法,我们可以使用类似于伪代码的方式来描述半加器电路的功能:
```vhdl
entity half_adder is
port(
a, b : in std_logic;
sum, carry : out std_logic
);
end half_adder;
architecture behavioral of half_adder is
begin
sum <= a xor b;
carry <= a and b;
end behavioral;
```
上面的代码中,我们定义了一个名为`half_adder`的实体,其中包含了两个输入端口`a`和`b`,以及两个输出端口`sum`和`carry`。在架构部分,我们使用逻辑门的真值表达式来描述半加器的功能。其中,`sum`输出的是输入`a`和`b`的异或结果,而`carry`输出的是输入`a`和`b`的与结果。
接下来是结构级描述方法,我们可以使用门级电路的方式来描述半加器电路的实现:
```vhdl
entity half_adder is
port(
a, b : in std_logic;
sum, carry : out std_logic
);
end half_adder;
architecture structural of half_adder is
signal and_gate_output : std_logic;
signal xor_gate_output : std_logic;
begin
and_gate_output <= a and b;
xor_gate_output <= a xor b;
sum <= xor_gate_output;
carry <= and_gate_output;
end structural;
```
上面的代码中,我们同样定义了一个名为`half_adder`的实体,其中包含了两个输入端口`a`和`b`,以及两个输出端口`sum`和`carry`。在架构部分,我们使用了两个信号`and_gate_output`和`xor_gate_output`来存储与门和异或门的输出结果。然后,我们将这两个信号的值分别赋给了`sum`和`carry`输出端口,完成了半加器电路的实现。
以上就是使用行为级和结构级两种描述方法来描述半加器电路的方法。希望能对您有所帮助。
vhdl行为描述设计半加器
VHDL(Very High-Speed Integrated Circuit Hardware Description Language)是一种硬件描述语言,用于系统级和电路级别的电子设计自动化(EDA)。对于行为描述设计,你可以使用VHDL编写一个简单的半加器,这是数字逻辑中最基础的组件之一,用于两个二进制位相加。
半加器的行为可以分为以下几个步骤:
1. **输入**:接受两个输入A和B以及一个“进位”输入Cin。其中A和B代表待加数的最低有效位,Cin是来自上一个位的进位信号。
2. **计算**:根据逻辑运算规则,如果当前位不需要进位(即两者都为0),则结果是A XOR B;如果需要进位,则结果是A AND B,并将Cout设置为1。
3. **输出**:生成两个输出,一个是本位的和(Sum),另一个是新的进位信号( Cout)。
以下是一个简化的VHDL半加器模块示例:
```vhdl
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
entity half_adder is
Port ( A, B : in STD_LOGIC;
Cin : in STD_LOGIC;
Sum : out STD_LOGIC;
Cout : out STD_LOGIC);
end half_adder;
architecture Behavioral of half_adder is
begin
Sum <= A xor B; -- 无进位时的和
Cout <= (A and B) or (not A and not B) and Cin; -- 计算进位
end Behavioral;
```
阅读全文
相关推荐
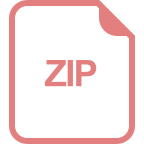
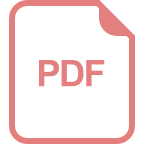
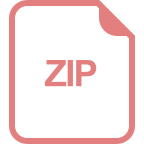
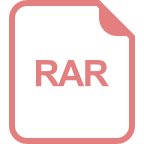
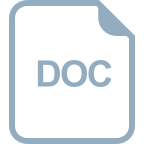
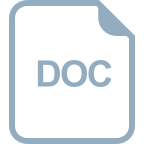
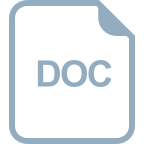
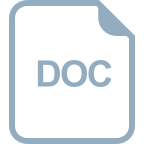
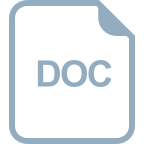


