使用pyhon调用sklearn库实现对鸢尾花数据的分类,采用逻辑回归分类方法,并计算以下多分类性能评价指标(两种方式):混淆矩阵、准确率、精确率、召回率、F1值、分类报告,并绘制PR曲线与ROC曲线。
时间: 2023-12-24 20:05:25 浏览: 84
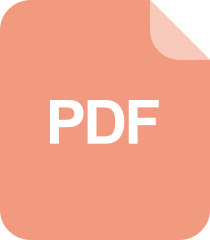
基于sklearn的logistic回归对于鸢尾花的机器学习分类实践

好的,以下是对于这个问题的解答:
首先,你需要先下载鸢尾花数据集,可以通过以下代码来获取:
```python
from sklearn.datasets import load_iris
iris = load_iris()
X, y = iris.data, iris.target
```
接着,你需要将数据集进行拆分,一部分用于训练模型,一部分用于测试模型:
```python
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
然后,你可以使用逻辑回归分类方法进行分类:
```python
from sklearn.linear_model import LogisticRegression
clf = LogisticRegression(random_state=42)
clf.fit(X_train, y_train)
y_pred = clf.predict(X_test)
```
接下来,你可以使用混淆矩阵、准确率、精确率、召回率、F1值、分类报告等指标来评估模型的性能:
```python
from sklearn.metrics import confusion_matrix, accuracy_score, precision_score, recall_score, f1_score, classification_report
print("Confusion matrix:\n", confusion_matrix(y_test, y_pred))
print("Accuracy:", accuracy_score(y_test, y_pred))
print("Precision:", precision_score(y_test, y_pred, average='weighted'))
print("Recall:", recall_score(y_test, y_pred, average='weighted'))
print("F1 score:", f1_score(y_test, y_pred, average='weighted'))
print("Classification report:\n", classification_report(y_test, y_pred))
```
最后,你可以使用PR曲线与ROC曲线来可视化模型的性能:
```python
import matplotlib.pyplot as plt
from sklearn.metrics import plot_precision_recall_curve, plot_roc_curve
plot_precision_recall_curve(clf, X_test, y_test)
plot_roc_curve(clf, X_test, y_test)
plt.show()
```
注意,由于鸢尾花数据集是一个3类分类问题,所以需要设置`average`参数为`'weighted'`,以计算加权平均值。如果你想计算微平均或宏平均,可以将`average`参数设置为`'micro'`或`'macro'`。
阅读全文
相关推荐
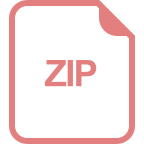
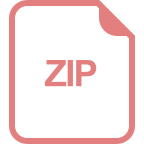
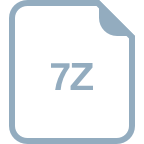
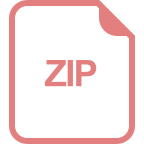
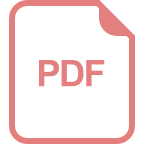
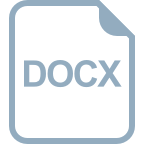









