用Android Studio软件编写微信界面
时间: 2023-11-15 12:49:55 浏览: 291
编写微信界面需要使用Android Studio软件,步骤如下:
1. 打开Android Studio软件,创建一个新的项目。
2. 在项目中创建布局文件,包括微信主界面、联系人列表、聊天列表和聊天界面等。
3. 创建适配器类来管理联系人列表和聊天列表,实现不同类型的联系人和聊天消息视图。
4. 在MainActivity中处理底部导航栏的点击事件,切换不同的Fragment。
5. 在联系人列表中,通过适配器显示不同的联系人信息,并实现搜索功能。
6. 在聊天列表中,通过适配器显示不同的聊天消息,并实现下拉刷新和上拉加载更多的功能。
7. 在聊天界面中,实现发送消息的逻辑,将发送的消息添加到聊天列表中。
具体实现过程可以参考以下教程:
1. [Android仿微信界面实现(一)底部导航栏](https://www.jianshu.com/p/5f0d57f7f201)
2. [Android仿微信界面实现(二)联系人列表](https://www.jianshu.com/p/3ef97ac78d9e)
3. [Android仿微信界面实现(三)聊天列表](https://www.jianshu.com/p/b9c2c3f5f26d)
4. [Android仿微信界面实现(四)聊天界面](https://www.jianshu.com/p/3e23e1d44bfc)
相关问题
用Android Studio编写微信界面
编写微信界面需要以下几个步骤:
1. 创建一个新的Android Studio项目并添加必要的依赖库。
2. 在布局文件中创建微信主界面,包括底部导航栏、联系人列表和聊天列表等。
3. 创建适配器类来管理联系人列表和聊天列表。
4. 在适配器中实现不同类型的联系人和聊天消息视图,例如文本、图片、语音等。
5. 在MainActivity中处理底部导航栏的点击事件,切换不同的Fragment。
6. 在联系人列表中,通过适配器显示不同的联系人信息,并实现搜索功能。
7. 在聊天列表中,通过适配器显示不同的聊天消息,并实现下拉刷新和上拉加载更多的功能。
8. 在聊天界面中,实现发送消息的逻辑,将发送的消息添加到聊天列表中。
具体实现过程可以参考以下教程:
1. [Android仿微信界面实现(一)底部导航栏](https://www.jianshu.com/p/5f0d57f7f201)
2. [Android仿微信界面实现(二)联系人列表](https://www.jianshu.com/p/3ef97ac78d9e)
3. [Android仿微信界面实现(三)聊天列表](https://www.jianshu.com/p/b9c2c3f5f26d)
4. [Android仿微信界面实现(四)聊天界面](https://www.jianshu.com/p/3e23e1d44bfc)
用Android Studio编写微信聊天界面
编写微信聊天界面需要以下几个步骤:
1. 创建一个新的Android Studio项目并添加必要的依赖库。
2. 在布局文件中创建聊天界面的UI,包括聊天消息列表、输入框和发送按钮等。
3. 创建一个适配器类来管理聊天消息列表。
4. 在适配器中实现不同类型的聊天消息视图,例如文本、图片、语音等。
5. 在MainActivity中处理发送消息的逻辑,将发送的消息添加到聊天消息列表中。
6. 在MainActivity中处理接收消息的逻辑,将接收到的消息添加到聊天消息列表中。
具体实现过程可以参考以下教程:
1. [Android仿微信聊天界面实现](https://www.jianshu.com/p/4e6edf0c3f25)
2. [Android仿微信聊天界面实现(二)](https://www.jianshu.com/p/cf2a7a1f312b)
3. [Android仿微信聊天界面实现(三)](https://www.jianshu.com/p/23b3f7d5d2f4)
阅读全文
相关推荐
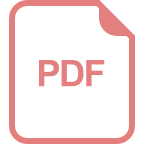
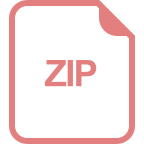
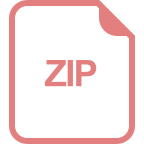
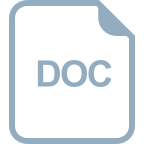


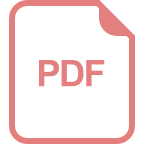
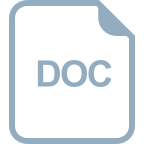
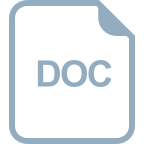
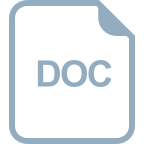
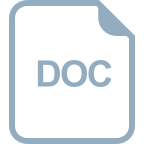



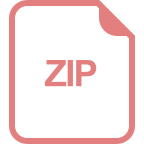