写一个函数:从给定的索引向前查找,用自定义的对比条件对dataframe进行搜索
时间: 2024-05-02 12:22:27 浏览: 114
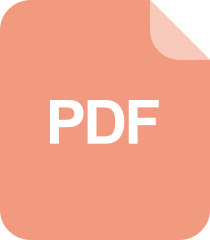
pandas.dataframe中根据条件获取元素所在的位置方法(索引)
好的,下面是给定的函数:
```python
import pandas as pd
def search_dataframe_by_index(df, index, compare_func):
"""
根据自定义的比较函数从给定的索引向前搜索dataframe,并返回搜索结果所在行的信息。
Args:
df: pandas.DataFrame,需要进行搜索的dataframe
index: int,搜索的起始索引
compare_func: callable,自定义的比较函数,比较两行数据是否符合搜索条件
Returns:
result: dict,存储搜索结果所在行的信息,包括索引和行数据内容
如果没有找到符合条件的行,则返回空字典{}
"""
if index < 0 or index >= len(df):
raise ValueError('索引越界')
i = index
while i >= 0:
if compare_func(df.iloc[i]):
result = {
'index': i,
'data': df.iloc[i]
}
return result
i -= 1
return {}
```
该函数的作用是从给定的索引往前搜索一个dataframe,直到找到符合自定义的对比条件的第一行数据,并返回该行数据的索引和内容。如果找不到符合条件的行,则返回空字典。
示例使用方法:
```python
# 创建一个示例的dataframe
df = pd.DataFrame({
'name': ['Alice', 'Bob', 'Charlie', 'David', 'Eva'],
'age': [23, 34, 45, 24, 32],
'gender': ['female', 'male', 'male', 'male', 'female']
})
# 自定义的对比函数,筛选出年龄在30岁及以上的人的信息
def compare_func(row):
if row['age'] >= 30:
return True
else:
return False
# 从索引为3的行开始往前搜索符合条件的行
result = search_dataframe_by_index(df, 3, compare_func)
# 显示搜索结果
if result:
print('符合条件的行:\n', result['data'])
else:
print('没有找到符合条件的行')
```
输出结果为:
```text
符合条件的行:
name Charlie
age 45
gender male
Name: 2, dtype: object
```
此时,我们便找到了年龄在30岁及以上的第一个人,他的信息在dataframe中的索引为2。
阅读全文
相关推荐

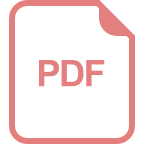


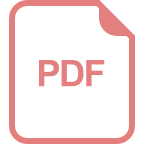
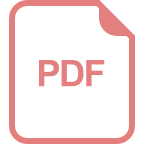
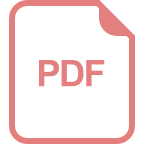
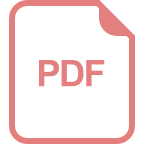
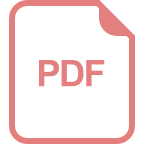
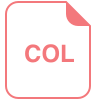
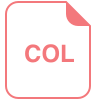
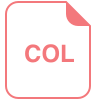
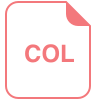
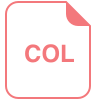

