Linux C 清空一个文件代码
时间: 2023-10-29 07:06:03 浏览: 94
清空一个文件可以使用 truncate 函数,其函数原型如下:
```c
#include <unistd.h>
int truncate(const char *path, off_t length);
```
其中,path 参数是要清空的文件路径,length 参数是要设置的文件大小。如果将 length 参数设置为 0,则可以清空整个文件。
下面是一个示例代码:
```c
#include <stdio.h>
#include <unistd.h>
int main() {
const char *path = "example.txt";
int result = truncate(path, 0);
if (result == -1) {
perror("truncate");
return 1;
}
printf("File %s has been cleared.\n", path);
return 0;
}
```
运行该程序后,文件 example.txt 中的内容就会被清空。
相关问题
linux如何用c语言清空一个文件
在 Linux 中,可以使用 C 语言的标准库函数 `fopen()` 和 `fclose()` 打开和关闭文件,使用 `ftruncate()` 函数清空一个文件。
下面是一个示例程序,展示如何清空一个文件:
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
int main() {
FILE *file = fopen("example.txt", "w");
if (file == NULL) {
perror("Failed to open file");
return 1;
}
if (ftruncate(fileno(file), 0) != 0) {
perror("Failed to truncate file");
fclose(file);
return 1;
}
fclose(file);
return 0;
}
```
在上面的示例程序中,首先使用 `fopen()` 函数打开一个文件,并指定以写入模式打开它。然后,使用 `fileno()` 函数获取文件描述符,将其作为第一个参数传递给 `ftruncate()` 函数,第二个参数传递为 0,表示将文件截断为 0 字节。最后,使用 `fclose()` 函数关闭文件。
Linux C 清空一个文件,并写入123,代码
以下是使用 C 语言在 Linux 系统中清空一个文件并写入 123 的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
int main() {
int fd = open("test.txt", O_WRONLY | O_TRUNC | O_CREAT, 0666);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
const char* data = "123";
size_t length = strlen(data);
ssize_t bytes_written = write(fd, data, length);
if (bytes_written == -1) {
perror("write");
exit(EXIT_FAILURE);
}
close(fd);
return 0;
}
```
其中 `open` 函数用于打开文件,`O_WRONLY` 表示以只写方式打开文件,`O_TRUNC` 表示清空文件内容,`O_CREAT` 表示如果文件不存在则创建该文件,`0666` 表示文件权限为读写。然后使用 `write` 函数向文件中写入 "123",最后关闭文件。
阅读全文
相关推荐
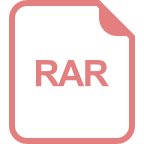


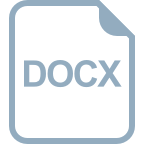
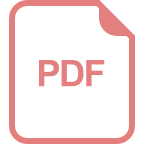
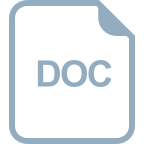
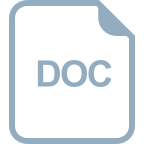






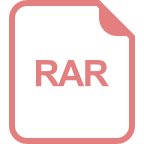
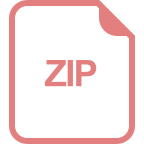