希尔排序和两种快速排序c语言
时间: 2023-06-22 12:25:29 浏览: 71
以下是希尔排序和两种快速排序的 C 语言实现代码:
### 希尔排序
```c
void shellSort(int arr[], int n) {
for (int gap = n/2; gap > 0; gap /= 2) {
for (int i = gap; i < n; i += 1) {
int temp = arr[i];
int j;
for (j = i; j >= gap && arr[j - gap] > temp; j -= gap) {
arr[j] = arr[j - gap];
}
arr[j] = temp;
}
}
}
```
### 快速排序
#### 递归实现
```c
void swap(int* a, int* b) {
int t = *a;
*a = *b;
*b = t;
}
int partition(int arr[], int low, int high) {
int pivot = arr[high];
int i = (low - 1);
for (int j = low; j <= high- 1; j++) {
if (arr[j] < pivot) {
i++;
swap(&arr[i], &arr[j]);
}
}
swap(&arr[i + 1], &arr[high]);
return (i + 1);
}
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
```
在这段代码中,`arr` 是待排序的数组,`low` 和 `high` 分别是数组的起始位置和结束位置。
首先,我们选择数组中的一个元素作为枢轴值(pivot),并将数组分为两部分,一部分小于枢轴值,一部分大于枢轴值。然后递归地对这两部分进行快速排序。
在 `partition` 函数中,我们选择数组最后一个元素作为枢轴值,然后遍历数组,将小于枢轴值的元素放到数组的左边,将大于枢轴值的元素放到数组的右边。最后,将枢轴值放到合适的位置,使得左边的元素都小于枢轴值,右边的元素都大于枢轴值。返回枢轴值的位置,即分割点。
在 `quickSort` 函数中,我们首先判断数组的长度是否大于 1,如果是,则选择一个枢轴值,将数组分为两部分,并递归地对这两部分进行快速排序。
#### 迭代实现
```c
void swap(int* a, int* b) {
int t = *a;
*a = *b;
*b = t;
}
void quickSort(int arr[], int n) {
int stack[n];
int top = -1;
stack[++top] = 0;
stack[++top] = n - 1;
while (top >= 0) {
int high = stack[top--];
int low = stack[top--];
int pivot = arr[high];
int i = (low - 1);
for (int j = low; j <= high- 1; j++) {
if (arr[j] < pivot) {
i++;
swap(&arr[i], &arr[j]);
}
}
swap(&arr[i + 1], &arr[high]);
int pi = (i + 1);
if (pi - 1 > low) {
stack[++top] = low;
stack[++top] = pi - 1;
}
if (pi + 1 < high) {
stack[++top] = pi + 1;
stack[++top] = high;
}
}
}
```
在这段代码中,我们使用一个栈来模拟递归实现的快速排序。首先,将整个数组的起始位置和结束位置压入栈中。然后,循环取出栈顶的两个位置,选择一个枢轴值,将数组分为两部分,并将子数组的起始位置和结束位置分别压入栈中。重复以上步骤,直到栈为空,排序完成。
这种迭代实现的快速排序算法称为非递归快速排序(Non-recursive QuickSort)。
相关推荐
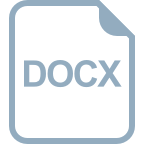
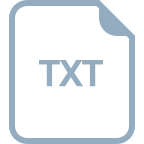
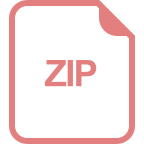














