java的复数类的实现及运算理论
时间: 2024-12-10 10:39:50 浏览: 17
在Java中,并没有内置的复数类,但我们可以自定义一个简单的复数类来进行基本的复数运算。一个常见的实现方式如下:
```java
public class ComplexNumber {
private double real;
private double imaginary;
public ComplexNumber(double real, double imaginary) {
this.real = real;
this.imaginary = imaginary;
}
// 获取实部和虚部
public double getReal() { return real; }
public double getImaginary() { return imaginary; }
// 设置复数值
public void setComplex(double real, double imaginary) {
this.real = real;
this.imaginary = imaginary;
}
// 复数运算
public ComplexNumber add(ComplexNumber other) {
double newReal = this.real + other.real;
double newImaginary = this.imaginary + other.imaginary;
return new ComplexNumber(newReal, newImaginary);
}
public ComplexNumber subtract(ComplexNumber other) {
double newReal = this.real - other.real;
double newImaginary = this.imaginary - other.imaginary;
return new ComplexNumber(newReal, newImaginary);
}
public ComplexNumber multiply(ComplexNumber other) {
double newReal = this.real * other.real - this.imaginary * other.imaginary;
double newImaginary = this.real * other.imaginary + this.imaginary * other.real;
return new ComplexNumber(newReal, newImaginary);
}
public ComplexNumber divide(ComplexNumber other) {
double denominator = Math.pow(other.real, 2) + Math.pow(other.imaginary, 2);
double newReal = (this.real * other.real + this.imaginary * other.imaginary) / denominator;
double newImaginary = (this.imaginary * other.real - this.real * other.imaginary) / denominator;
return new ComplexNumber(newReal, newImaginary);
}
// 返回复数字符串表示形式
@Override
public String toString() {
return "(" + real + " + " + imaginary + "i)";
}
}
```
在这个例子中,我们实现了加法、减法、乘法和除法运算,以及将复数转换为字符串的方法。复数的运算是基于数学中的笛卡尔坐标系统,遵循标准的代数运算规则。
阅读全文
相关推荐
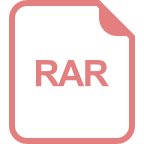

















