用c++写一个带密码和职位的员工工资管理系统
时间: 2024-05-07 11:20:25 浏览: 95
好的,下面是一个简单的员工工资管理系统的代码示例:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
// 定义Employee类
class Employee {
private:
string name;
string password;
string position;
double salary;
public:
Employee(string n, string p, string pos, double s) {
name = n;
password = p;
position = pos;
salary = s;
}
string getName() const {
return name;
}
string getPassword() const {
return password;
}
string getPosition() const {
return position;
}
double getSalary() const {
return salary;
}
void setPassword(string p) {
password = p;
}
void setPosition(string pos) {
position = pos;
}
void setSalary(double s) {
salary = s;
}
// 重载运算符,用于比较Employee对象的大小
bool operator<(const Employee& e) const {
return name < e.getName();
}
// 友元函数,用于在屏幕上输出Employee对象的信息
friend ostream& operator<<(ostream& os, const Employee& e);
};
// 在屏幕上输出Employee对象的信息
ostream& operator<<(ostream& os, const Employee& e) {
os << "Name: " << e.name << ", Position: " << e.position << ", Salary: " << e.salary;
return os;
}
// 读取员工信息文件
vector<Employee> readEmployees(string filename) {
vector<Employee> employees;
ifstream infile(filename);
if (!infile.is_open()) {
cerr << "Failed to open file: " << filename << endl;
return employees;
}
string name, password, position;
double salary;
while (infile >> name >> password >> position >> salary) {
Employee e(name, password, position, salary);
employees.push_back(e);
}
infile.close();
return employees;
}
// 写入员工信息文件
void writeEmployees(string filename, const vector<Employee>& employees) {
ofstream outfile(filename);
if (!outfile.is_open()) {
cerr << "Failed to open file: " << filename << endl;
return;
}
for (const Employee& e : employees) {
outfile << e.getName() << " " << e.getPassword() << " " << e.getPosition() << " " << e.getSalary() << endl;
}
outfile.close();
}
// 添加员工信息
void addEmployee(vector<Employee>& employees) {
string name, password, position;
double salary;
cout << "Enter name: ";
cin >> name;
cout << "Enter password: ";
cin >> password;
cout << "Enter position: ";
cin >> position;
cout << "Enter salary: ";
cin >> salary;
Employee e(name, password, position, salary);
employees.push_back(e);
cout << "Employee added successfully." << endl;
}
// 删除员工信息
void deleteEmployee(vector<Employee>& employees) {
string name;
cout << "Enter name of employee to delete: ";
cin >> name;
for (auto it = employees.begin(); it != employees.end(); ++it) {
if (it->getName() == name) {
employees.erase(it);
cout << "Employee deleted successfully." << endl;
return;
}
}
cout << "Employee not found." << endl;
}
// 修改员工信息
void modifyEmployee(vector<Employee>& employees) {
string name;
cout << "Enter name of employee to modify: ";
cin >> name;
for (auto& e : employees) {
if (e.getName() == name) {
string password, position;
double salary;
cout << "Enter new password: ";
cin >> password;
cout << "Enter new position: ";
cin >> position;
cout << "Enter new salary: ";
cin >> salary;
e.setPassword(password);
e.setPosition(position);
e.setSalary(salary);
cout << "Employee modified successfully." << endl;
return;
}
}
cout << "Employee not found." << endl;
}
// 查找员工信息
void findEmployee(const vector<Employee>& employees) {
string name;
cout << "Enter name of employee to find: ";
cin >> name;
for (const auto& e : employees) {
if (e.getName() == name) {
cout << e << endl;
return;
}
}
cout << "Employee not found." << endl;
}
// 显示所有员工信息
void displayEmployees(const vector<Employee>& employees) {
for (const auto& e : employees) {
cout << e << endl;
}
}
// 根据员工名字排序并显示
void sortEmployees(vector<Employee>& employees) {
sort(employees.begin(), employees.end());
displayEmployees(employees);
}
int main() {
string password;
cout << "Enter password to access the system: ";
cin >> password;
if (password != "password") {
cout << "Access denied." << endl;
return 0;
}
vector<Employee> employees = readEmployees("employees.txt");
if (employees.empty()) {
cout << "No employee data found." << endl;
return 0;
}
int choice;
do {
cout << endl;
cout << "1. Add employee" << endl;
cout << "2. Delete employee" << endl;
cout << "3. Modify employee" << endl;
cout << "4. Find employee" << endl;
cout << "5. Display all employees" << endl;
cout << "6. Sort employees by name" << endl;
cout << "0. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1:
addEmployee(employees);
writeEmployees("employees.txt", employees);
break;
case 2:
deleteEmployee(employees);
writeEmployees("employees.txt", employees);
break;
case 3:
modifyEmployee(employees);
writeEmployees("employees.txt", employees);
break;
case 4:
findEmployee(employees);
break;
case 5:
displayEmployees(employees);
break;
case 6:
sortEmployees(employees);
break;
case 0:
writeEmployees("employees.txt", employees);
break;
default:
cout << "Invalid choice." << endl;
break;
}
} while (choice != 0);
return 0;
}
```
这个代码示例实现了以下功能:
1. 在程序运行前,需要输入密码才能进入系统。
2. 程序从文件 "employees.txt" 中读取员工信息,然后将它们存储在一个 vector<Employee> 对象中。
3. 程序提供了以下选项:
1. 添加员工信息。
2. 删除员工信息。
3. 修改员工信息。
4. 查找员工信息。
5. 显示所有员工信息。
6. 根据员工名字排序并显示。
7. 退出程序。
4. 每次进行修改、删除、添加员工信息操作后,程序都将更新员工信息文件 "employees.txt"。
请注意,这个代码示例仅仅是一个简单的员工工资管理系统,它还有很多可以改进的地方。比如,它没有对用户输入进行错误处理,也没有对文件读写操作进行异常处理。在实际应用中,这些问题都需要得到仔细处理。同时,还需要考虑如何保护敏感信息(比如密码)的安全性。
阅读全文
相关推荐
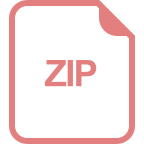
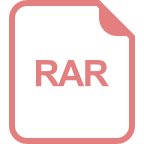
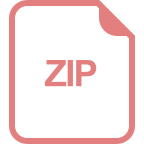















