假设有两个进程p1和p2,这两个进程在他们并发执行的某一时刻排它的访问一个共享 资源。可定义一个信号量s,其初始值为1,并且可以为两个进程所访问。两个进程需要执 行同样的处理来访问临界区代码,请试着编写程序完成上述要求。 提示:这两个进程可以是同一个程序的两次调用。c代码并注释
时间: 2024-11-30 07:16:21 浏览: 11
为了实现这个场景,我们可以使用互斥锁(mutex)来控制对共享资源的并发访问。在C语言中,通常使用`pthread_mutex_t`来创建互斥锁。以下是简单的C代码示例:
```c
#include <stdio.h>
#include <pthread.h>
// 初始化互斥锁
pthread_mutex_t critical_section_mutex = PTHREAD_MUTEX_INITIALIZER;
// 这是我们要保护的临界区代码
void critical_section(void* arg) {
pthread_mutex_lock(&critical_section_mutex); // 获取互斥锁
printf("Process %d entered critical section\n", pthread_self());
// 进入临界区的操作...
// ...
pthread_mutex_unlock(&critical_section_mutex); // 释放互斥锁
}
int main() {
// 创建两个线程,分别代表进程p1和p2
pthread_t thread1, thread2;
int result;
// 线程1
if ((result = pthread_create(&thread1, NULL, critical_section, NULL)) != 0) {
printf("Error creating thread 1: %d\n", result);
return -1;
}
// 线程2
if ((result = pthread_create(&thread2, NULL, critical_section, NULL)) != 0) {
printf("Error creating thread 2: %d\n", result);
pthread_cancel(thread1); // 如果创建失败,尝试取消第一个线程
return -1;
}
// 等待两个线程结束
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
printf("Both threads exited normally\n");
return 0;
}
```
在这个例子中,`pthread_mutex_lock`用于获取互斥锁,进入临界区;`pthread_mutex_unlock`用于释放互斥锁,允许其他线程访问。这样就保证了任何时候只有一个进程能够进入临界区。
阅读全文
相关推荐
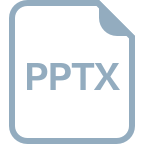
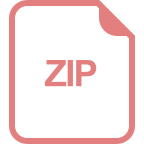
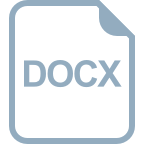
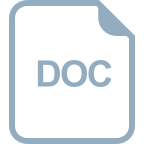
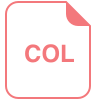
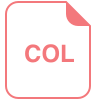
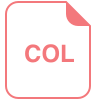
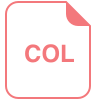
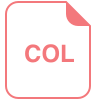
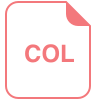
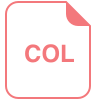
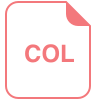
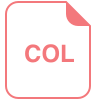
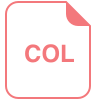
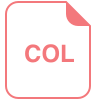
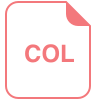
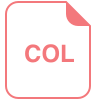
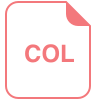
