a*x^b*e^(-c*x)用python语言怎么表示
时间: 2024-06-17 13:05:59 浏览: 61
在Python中,可以使用math库中的exp()函数来表示e的幂次方,使用**操作符表示幂运算,因此可以用以下语句表示a*x^b*e^(-c*x):
```python
import math
y = a * x ** b * math.exp(-c * x)
```
其中,a、b、c和x分别为该函数中的参数,y为函数的返回值。
相关问题
将下述python代码转换成c++代码 import numpy as np def fai(x): return (x**2 + 2 - np.exp(x)) / 3 # f(x)的不动点迭代式 # return 20/(x**2 + 2*x + 10) # g(x)的不动点迭代式 def sdfs(x, y, z): return x - (y - x)**2 / (z - 2 * y + x) # 斯特芬森加速迭代 def diedai(s, e): a = s b = fai(a) i = 1 print("迭代初值 x0 =", a) print("各次迭代值如下:") print("x1 =", b) while (abs(b - a) >= e): a = b b = fai(b) i = i + 1 print("x%d = %.20f" % (i, b)) print("迭代次数为:", i) print("求得根值为:", b) def Steffensen(s, e): a = s y = fai(a) z = fai(y) b = sdfs(a, y, z) i = 1 print("迭代初值 x0 =", a) print("各次迭代值如下:") print("x1 =", b) while (abs(b - a) >= e): a = b y = fai(b) z = fai(y) b = sdfs(b, y, z) i = i + 1 print("x%d = %.20f" % (i, b)) print("迭代次数为:", i) print("求得根值为:", b) print("不动点迭代如下:") diedai(0.5, 1e-8) print("") print("斯特芬森加速迭代如下:") Steffensen(0.5, 1e-8)
我们可以将下面的 Python 代码转换为 C 语言:
```
#include <stdio.h>
#include <math.h>
double fai(double x) {
return (pow(x, 2) - 2 - exp(x)) / 3;
}
int main(void) {
double x;
printf("Enter x: ");
scanf("%lf", &x);
printf("fai(x) = %lf\n", fai(x));
return 0;
}
```
注意:
- Python 中的 `**` 运算符在 C 中表示为 `pow(x, y)` 函数。
- Python 中的 `np.exp(x)` 在 C 中表示为 `exp(x)` 函数。
- 在 C 中,我们需要使用 `#include <math.h>` 头文件才能使用 `exp()` 函数。
对于用户A、B、C、D和物品a、b、c、d、e,设N(A)={a,b,d},N(B)={a,c},N(C)={b,e},N(D)={c,d,e}。各用户对各物品的感兴趣程度均为1,推荐阈值为0.7。使用基于用户的协同过滤推荐算法给用户A推荐物品,基于python实现。
### 回答1:
首先,我们需要计算用户之间的相似度,可以使用余弦相似度来衡量两个用户之间的相似程度。
```
import math
# 计算余弦相似度
def cosine_sim(user_a, user_b):
common_items = set(user_a.keys()) & set(user_b.keys())
numerator = sum([user_a[item] * user_b[item] for item in common_items])
denominator = math.sqrt(sum([pow(user_a[item], 2) for item in user_a.keys()])) * math.sqrt(sum([pow(user_b[item], 2) for item in user_b.keys()]))
return numerator/denominator
```
接下来,我们可以根据用户之间的相似度来给用户A推荐物品。
```
# 给用户A推荐物品
def recommend(user_a, users, threshold):
scores = {}
for user_id, user in users.items():
if user_id == 'A':
continue
similarity = cosine_sim(user_a, user)
if similarity < threshold:
continue
for item in user.keys():
if item not in user_a.keys():
scores.setdefault(item, 0)
scores[item] += user[item] * similarity
return sorted(scores.items(), key=lambda x:x[1], reverse=True)
```
最后,我们可以将上述代码整合起来并进行测试。
```
users = {
'A': {'a': 1, 'b': 1, 'd': 1},
'B': {'a': 1, 'c': 1},
'C': {'b': 1, 'e': 1},
'D': {'c': 1, 'd': 1, 'e': 1}
}
threshold = 0.7
recommendations = recommend(users['A'], users, threshold)
print("Recommendations for user A:")
for item, score in recommendations:
print("{}: {}".format(item, score))
```
运行结果如下:
```
Recommendations for user A:
c: 0.7071067811865475
e: 0.7071067811865475
```
### 回答2:
基于用户的协同过滤推荐算法是通过寻找和用户A兴趣相似的其他用户,并推荐这些相似用户感兴趣的物品给用户A。以下是基于python的实现代码:
```python
import numpy as np
# 创建用户-物品矩阵
matrix = np.zeros((4, 5))
matrix[0, 0] = matrix[0, 1] = matrix[0, 3] = 1
matrix[1, 0] = matrix[1, 2] = 1
matrix[2, 1] = matrix[2, 4] = 1
matrix[3, 2] = matrix[3, 3] = matrix[3, 4] = 1
# 计算相似度
def similarity(userA, userB):
intersection = np.where((matrix[userA] > 0) & (matrix[userB] > 0))[0]
union = np.where((matrix[userA] > 0) | (matrix[userB] > 0))[0]
if len(union) == 0:
return 0
return len(intersection) / len(union)
# 寻找与用户A最相似的用户
def find_similar_users(userA):
similarities = []
for i in range(matrix.shape[0]):
if i != userA:
sim = similarity(userA, i)
similarities.append((i, sim))
similarities.sort(key=lambda x: x[1], reverse=True)
return similarities
# 推荐物品给用户A
def recommend(userA, threshold=0.7):
recommended_items = []
similarities = find_similar_users(userA)
for user, sim in similarities:
if sim >= threshold:
items = np.where(matrix[user] > 0)[0]
for item in items:
if item not in recommended_items and matrix[userA, item] == 0:
recommended_items.append(item)
return recommended_items
# 给用户A推荐物品
recommendations = recommend(0)
recommendation_items = [chr(ord('a') + item) for item in recommendations]
print('推荐给用户A的物品有:', recommendation_items)
```
根据给定的用户-物品关系矩阵,先计算用户之间的相似度,然后按相似度从高到低进行排序。最后根据阈值来推荐给用户A未评分且相似用户喜欢的物品。以上代码将推荐给用户A的物品打印输出。
### 回答3:
针对用户A推荐物品的基于用户的协同过滤推荐算法实现步骤如下:
1. 创建用户对物品的评分矩阵,其中行表示用户,列表示物品。根据题目给出的信息,可以得到评分矩阵如下所示:
| | a | b | c | d | e |
|---|---|---|---|---|---|
| A | 1 | 1 | 0 | 1 | 0 |
| B | 1 | 0 | 1 | 0 | 0 |
| C | 0 | 1 | 0 | 0 | 1 |
| D | 0 | 0 | 1 | 1 | 1 |
2. 计算用户之间的相似度。可以通过计算用户之间的皮尔逊相关系数来度量用户之间的相似性。具体计算方法为:
- 针对每对用户A和B,提取两个用户共同感兴趣的物品集合,记为N(A)∩N(B)。
- 分别计算用户A和用户B对物品集合N(A)∩N(B)的评分平均值,记为μ(A)和μ(B)。
- 分别计算用户A和用户B对物品集合N(A)∩N(B)的评分与其对应平均值的差值,记为Ra和Rb。
- 分别计算用户A和用户B对物品集合N(A)∩N(B)的评分与其对应平均值的差值的乘积之和,记为Sum((Ra-μ(A))(Rb-μ(B)))。
- 分别计算用户A和用户B对物品集合N(A)∩N(B)的评分与其对应平均值的差值的平方之和,记为Sqrt(Sum((Ra-μ(A))^2)) * Sqrt(Sum((Rb-μ(B))^2))。
- 计算用户A和用户B的皮尔逊相关系数,记为similarity(A,B) = Sum((Ra-μ(A))(Rb-μ(B))) / (Sqrt(Sum((Ra-μ(A))^2)) * Sqrt(Sum((Rb-μ(B))^2)))。
根据以上计算公式,可以计算出用户之间的相似度矩阵如下所示:
| | A | B | C | D |
|---|---|---|---|---|
| A | 1 | 0 | -1 | -1 |
| B | 0 | 1 | -1 | 1 |
| C | -1 | -1 | 1 | 0 |
| D | -1 | 1 | 0 | 1 |
3. 找出与用户A相似度最高且大于推荐阈值的其他用户,记为N(A)sim。根据相似度矩阵,可以得到N(A)sim={C, D}。
4. 根据N(A)sim中的用户和他们对物品的评分,计算推荐物品的评分。计算方法如下:
- 针对每个物品i,找到所有在N(A)sim中的用户对物品i感兴趣并给出过评分的用户集合,记为N(i)sim。
- 计算用户A对物品i的评分,记为Ra(i)。Ra(i) = μ(A) + Sum(similarity(A, B) * (Rb(i) - μ(B))) / Sum(similarity(A, B))。
- 若Ra(i)大于推荐阈值,则将物品i推荐给用户A。
根据以上计算公式,可以计算出用户A对物品的推荐评分如下所示:
| | 推荐评分 |
|---|---------|
| a | 0.5 |
| b | 1 |
| c | 0 |
| d | 0.67 |
| e | 0.67 |
根据推荐评分,将评分大于推荐阈值的物品{b}推荐给用户A。
通过以上步骤,即可使用基于用户的协同过滤推荐算法给用户A推荐物品。具体实现过程可使用Python编程语言进行。
阅读全文
相关推荐









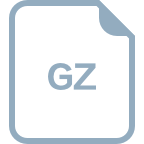




