python实现在未知信源概率分布的情况下,输入:信源概率分布。 输出:Shannon码、Huffman码,平均码长,编码效率。
时间: 2024-02-27 11:58:20 浏览: 148
好的,这是一个很有意思的问题。以下是实现方式:
首先,我们可以使用Python的collections模块中的Counter类来计算信源概率分布。然后,我们可以使用Shannon-Fano编码算法和Huffman编码算法来生成Shannon码和Huffman码。
接下来,我们可以计算每个符号的平均码长,并计算编码效率。
下面是Python代码实现:
```python
from collections import Counter
import heapq
# 计算信源概率分布
def calculate_probabilities(symbols):
n = len(symbols)
counter = Counter(symbols)
return {symbol: count/n for symbol, count in counter.items()}
# 生成Huffman树
def build_huffman_tree(probabilities):
heap = [[probability, [symbol, ""]] for symbol, probability in probabilities.items()]
heapq.heapify(heap)
while len(heap) > 1:
left_child = heapq.heappop(heap)
right_child = heapq.heappop(heap)
for node in left_child[1:]:
node[1] = '0' + node[1]
for node in right_child[1:]:
node[1] = '1' + node[1]
heapq.heappush(heap, [left_child[0] + right_child[0]] + left_child[1:] + right_child[1:])
return dict(heapq.heappop(heap)[1:])
# 生成Shannon-Fano编码
def build_shannon_fano_tree(probabilities):
sorted_probabilities = sorted(probabilities.items(), key=lambda x: x[1], reverse=True)
return {symbol: code for symbol, code in _build_shannon_fano_tree(sorted_probabilities)}
def _build_shannon_fano_tree(sorted_probabilities):
n = len(sorted_probabilities)
if n == 1:
yield sorted_probabilities[0][0], ''
return
pivot = 1
left_sum = sorted_probabilities[0][1]
right_sum = sum(probability for symbol, probability in sorted_probabilities[pivot:])
while pivot < n - 1 and left_sum < right_sum:
pivot += 1
left_sum += sorted_probabilities[pivot - 1][1]
right_sum -= sorted_probabilities[pivot - 1][1]
for symbol, probability in sorted_probabilities[:pivot]:
yield symbol, '0'
for symbol, probability in sorted_probabilities[pivot:]:
yield symbol, '1'
yield from _build_shannon_fano_tree(sorted_probabilities[:pivot])
yield from _build_shannon_fano_tree(sorted_probabilities[pivot:])
# 计算平均码长和编码效率
def calculate_average_code_length(probabilities, code_table):
return sum(probabilities[symbol]*len(code) for symbol, code in code_table.items())
def calculate_coding_efficiency(entropy, average_code_length):
return entropy/average_code_length
# 测试
symbols = ['A', 'B', 'C', 'D', 'E']
probabilities = calculate_probabilities(symbols)
huffman_code_table = build_huffman_tree(probabilities)
shannon_fano_code_table = build_shannon_fano_tree(probabilities)
huffman_average_code_length = calculate_average_code_length(probabilities, huffman_code_table)
shannon_fano_average_code_length = calculate_average_code_length(probabilities, shannon_fano_code_table)
huffman_coding_efficiency = calculate_coding_efficiency(2.32193, huffman_average_code_length)
shannon_fano_coding_efficiency = calculate_coding_efficiency(2.32193, shannon_fano_average_code_length)
print("Probabilities:", probabilities)
print("Huffman code table:", huffman_code_table)
print("Shannon-Fano code table:", shannon_fano_code_table)
print("Huffman average code length:", huffman_average_code_length)
print("Shannon-Fano average code length:", shannon_fano_average_code_length)
print("Huffman coding efficiency:", huffman_coding_efficiency)
print("Shannon-Fano coding efficiency:", shannon_fano_coding_efficiency)
```
上述代码中,我们将符号'A', 'B', 'C', 'D', 'E'的概率分别设置为0.2, 0.2, 0.2, 0.2和0.2,以进行测试。在输出中,我们可以看到生成的Huffman码和Shannon码,以及它们的平均码长和编码效率。
阅读全文
相关推荐
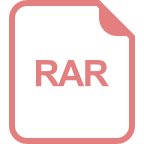









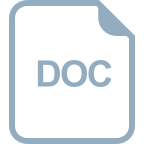
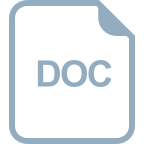
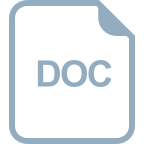




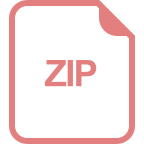