写一段Java代码实现map
时间: 2023-09-20 08:06:13 浏览: 72
### 回答1:
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<String, Integer>();
map.put("A", 1);
map.put("B", 2);
map.put("C", 3);
map.forEach((key, value) -> System.out.println(key + " " + value));
}
### 回答2:
请问您想要实现的是什么类型的map呢?是HashMap还是TreeMap呢?以下是一个简单的示例代码实现HashMap的方式:
```java
import java.util.HashMap;
import java.util.Map;
public class MyMap {
public static void main(String[] args) {
// 创建一个HashMap对象
Map<String, Integer> myMap = new HashMap<>();
// 向map中添加键值对
myMap.put("张三", 18);
myMap.put("李四", 20);
myMap.put("王五", 22);
// 获取map中的值
int age = myMap.get("张三");
System.out.println("张三的年龄是:" + age);
// 判断map中是否包含指定的键或值
boolean containsKey = myMap.containsKey("李四");
boolean containsValue = myMap.containsValue(25);
System.out.println("map中是否包含李四:" + containsKey);
System.out.println("map中是否包含值为25的键值对:" + containsValue);
// 遍历map中的键值对
for (Map.Entry<String, Integer> entry : myMap.entrySet()) {
String key = entry.getKey();
int value = entry.getValue();
System.out.println("姓名:" + key + ",年龄:" + value);
}
// 修改map中的值
myMap.put("王五", 23);
System.out.println("修改后王五的年龄是:" + myMap.get("王五"));
// 删除map中的键值对
myMap.remove("张三");
System.out.println("删除后张三的年龄是:" + myMap.get("张三"));
}
}
```
### 回答3:
实现Map的常用方式是使用Java提供的HashMap类。下面是一个简单的示例代码:
```java
import java.util.HashMap;
import java.util.Map;
public class MyMap {
public static void main(String[] args) {
// 创建一个HashMap实例
Map<String, Integer> map = new HashMap<>();
// 向map中添加键值对
map.put("apple", 1);
map.put("banana", 2);
map.put("orange", 3);
// 获取map的大小
System.out.println("Map size: " + map.size());
// 判断map是否包含某个键或值
System.out.println("Contains key 'apple': " + map.containsKey("apple"));
System.out.println("Contains value 3: " + map.containsValue(3));
// 获取map中的某个值
System.out.println("Value for key 'banana': " + map.get("banana"));
// 遍历map的键值对
for (Map.Entry<String, Integer> entry : map.entrySet()) {
System.out.println(entry.getKey() + " : " + entry.getValue());
}
// 移除map中的某个键值对
map.remove("orange");
// 清空map
map.clear();
// 判断map是否为空
System.out.println("Is map empty: " + map.isEmpty());
}
}
```
这段示例代码演示了如何创建一个HashMap实例,向其中添加键值对,获取map的大小、判断是否包含某个键或值、获取某个键对应的值、遍历map的键值对等操作。同时也展示了如何移除map中的某个键值对,清空map,以及判断map是否为空。
相关推荐
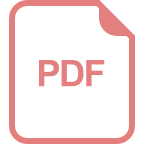
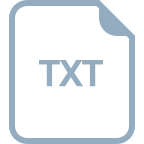
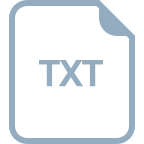
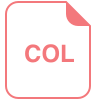













