c# GDI 带角度的矩形鼠标改变缩放矩形大小
时间: 2024-04-20 12:27:04 浏览: 180
您可以通过以下步骤实现带角度的矩形鼠标改变缩放矩形大小:
1. 定义一个类来表示带角度的矩形,该类应包含矩形的位置、大小和角度等信息。
2. 在矩形的周围绘制一些调整大小的手柄,使用户可以使用鼠标拖拽来调整矩形的大小。
3. 当用户拖拽调整大小的手柄时,根据手柄的位置和移动距离来计算矩形的新位置和大小,并重新绘制矩形。
4. 当用户拖拽矩形本身时,根据鼠标的移动距离来计算矩形的新位置,并重新绘制矩形。
5. 当用户拖拽矩形的角度调整手柄时,根据鼠标的移动距离来计算矩形的新角度,并重新绘制矩形。
6. 为了实现缩放时的平移效果,可以在拖拽调整大小的手柄时,根据手柄的位置和移动距离来计算缩放中心点,并将矩形的位置相应地调整。
注意:实现带角度的矩形鼠标改变缩放矩形大小涉及到一些复杂的数学计算,需要仔细考虑并实现相应的算法。
阅读全文
相关推荐




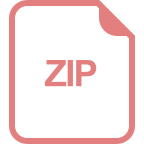


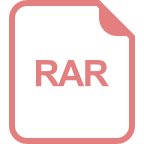



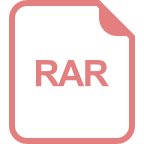


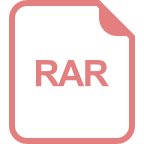

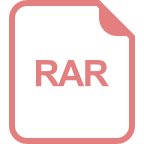

