springboot离线地理位置解析代码
时间: 2023-07-03 16:26:56 浏览: 88
以下是一个简单的 Spring Boot 离线地理位置解析的代码示例:
1. 首先,需要将离线地理位置数据(例如 OpenStreetMap 数据)下载到本地存储。
2. 在 Spring Boot 项目的 pom.xml 文件中添加以下依赖:
```xml
<dependency>
<groupId>org.locationtech.jts</groupId>
<artifactId>jts-core</artifactId>
<version>1.16.0</version>
</dependency>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-csv</artifactId>
<version>1.8</version>
</dependency>
```
3. 创建一个 GeoUtils 工具类,使用 JTS 库读取离线地理位置数据,并提供一个方法来根据经纬度坐标获取地址信息。以下是该类的示例代码:
```java
import java.io.File;
import java.io.IOException;
import java.nio.charset.Charset;
import java.util.List;
import org.apache.commons.csv.CSVFormat;
import org.apache.commons.csv.CSVParser;
import org.apache.commons.csv.CSVRecord;
import org.locationtech.jts.geom.Geometry;
import org.locationtech.jts.geom.Point;
import org.locationtech.jts.index.strtree.STRtree;
import org.springframework.util.ResourceUtils;
public class GeoUtils {
private static final String DATA_FILE = "classpath:data/cities.csv";
private static final int GEOMETRY_COLUMN = 0;
private static final int NAME_COLUMN = 1;
private static final int COUNTRY_COLUMN = 8;
private static final Charset CHARSET = Charset.forName("UTF-8");
private static final STRtree INDEX = new STRtree();
static {
try {
File file = ResourceUtils.getFile(DATA_FILE);
CSVParser parser = CSVParser.parse(file, CHARSET, CSVFormat.DEFAULT);
List<CSVRecord> records = parser.getRecords();
for (CSVRecord record : records) {
String wkt = record.get(GEOMETRY_COLUMN);
String name = record.get(NAME_COLUMN);
String country = record.get(COUNTRY_COLUMN);
Geometry geometry = JTSUtils.read(wkt);
City city = new City(name, country, geometry);
INDEX.insert(geometry.getEnvelopeInternal(), city);
}
INDEX.build();
} catch (IOException e) {
e.printStackTrace();
}
}
public static String getAddress(double longitude, double latitude) {
Point point = JTSUtils.createPoint(longitude, latitude);
List<?> cities = INDEX.query(point.getEnvelopeInternal());
for (Object obj : cities) {
City city = (City) obj;
if (city.getGeometry().contains(point)) {
return city.getName() + ", " + city.getCountry();
}
}
return null;
}
private static class City {
private String name;
private String country;
private Geometry geometry;
public City(String name, String country, Geometry geometry) {
this.name = name;
this.country = country;
this.geometry = geometry;
}
public String getName() {
return name;
}
public String getCountry() {
return country;
}
public Geometry getGeometry() {
return geometry;
}
}
}
```
4. 在 Spring Boot 控制器中使用 GeoUtils 工具类,调用 getAddress 方法获取地址信息。以下是该控制器的示例代码:
```java
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class GeoController {
@GetMapping("/address")
public String getAddress(@RequestParam double longitude, @RequestParam double latitude) {
String address = GeoUtils.getAddress(longitude, latitude);
if (address != null) {
return address;
} else {
return "Unknown";
}
}
}
```
上述代码示例中,我们使用 JTS 库读取了一个包含城市位置信息的 CSV 文件,并将它们插入到一个 STRtree 索引中。当调用 getAddress 方法时,我们使用 JTS 库创建一个 Point 对象表示经纬度坐标,并在 STRtree 索引中查找该点所在的城市。如果找到了城市,则返回城市名称和国家名,否则返回 Unknown。
相关推荐
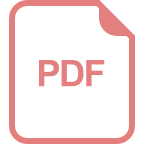
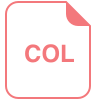
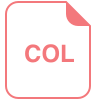
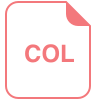
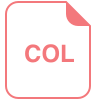
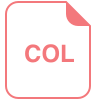









