将 G711a 数据帧转换为 PCM 数据帧,C++示例
时间: 2024-02-21 22:59:14 浏览: 27
将 G711a 数据帧转换为 PCM 数据帧的过程其实就是解码过程,可以使用 FFmpeg 中的 libavcodec 库进行实现。下面是一个简单的示例代码:
```cpp
#include <iostream>
#include <cstring>
#include "libavcodec/avcodec.h"
int main() {
// Initialize FFmpeg
av_register_all();
avcodec_register_all();
// Allocate a G711a decoder context
AVCodec* codec = avcodec_find_decoder(AV_CODEC_ID_PCM_ALAW);
AVCodecContext* codecCtx = avcodec_alloc_context3(codec);
avcodec_open2(codecCtx, codec, NULL);
// Allocate a PCM decoder context
AVCodec* pcmCodec = avcodec_find_decoder(AV_CODEC_ID_PCM_S16LE);
AVCodecContext* pcmCodecCtx = avcodec_alloc_context3(pcmCodec);
avcodec_open2(pcmCodecCtx, pcmCodec, NULL);
// G711a data frame
uint8_t g711aData[] = {0x74, 0x1c, 0x1a, 0x1d, 0x1a, 0x1d, 0x1c, 0x1d};
int g711aDataSize = sizeof(g711aData);
// Allocate a packet and a frame for G711a data
AVPacket* pkt = av_packet_alloc();
av_new_packet(pkt, g711aDataSize);
memcpy(pkt->data, g711aData, g711aDataSize);
AVFrame* frame = av_frame_alloc();
// Decode G711a data to PCM data
int ret = avcodec_send_packet(codecCtx, pkt);
if (ret >= 0) {
ret = avcodec_receive_frame(codecCtx, frame);
if (ret >= 0) {
// Allocate a packet and a frame for PCM data
AVPacket* pcmPkt = av_packet_alloc();
AVFrame* pcmFrame = av_frame_alloc();
// Resample the PCM data to 8kHz and mono channel
SwrContext* swrCtx = swr_alloc_set_opts(NULL,
av_get_default_channel_layout(1),
AV_SAMPLE_FMT_S16,
8000,
av_get_default_channel_layout(codecCtx->channels),
codecCtx->sample_fmt,
codecCtx->sample_rate,
0,
NULL);
swr_init(swrCtx);
// Decode the PCM data
ret = avcodec_send_frame(pcmCodecCtx, pcmFrame);
if (ret >= 0) {
ret = avcodec_receive_packet(pcmCodecCtx, pcmPkt);
if (ret >= 0) {
// pcmPkt->data contains the PCM data
std::cout << "PCM data size: " << pcmPkt->size << std::endl;
}
}
// Free resources
av_packet_free(&pcmPkt);
av_frame_free(&pcmFrame);
swr_free(&swrCtx);
}
}
// Free resources
av_packet_free(&pkt);
av_frame_free(&frame);
avcodec_free_context(&codecCtx);
avcodec_free_context(&pcmCodecCtx);
return 0;
}
```
上面的代码将一个 G711a 数据帧解码为 PCM 数据帧,并将 PCM 数据帧进行重采样,最终得到的 PCM 数据是 8kHz 的采样率和单声道的。具体的重采样过程可以参考 FFmpeg 中的 libswresample 库的相关函数。
相关推荐
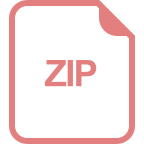














