jt1078协议的G711a音频帧数据,用ffmpeg推流,C++实现
时间: 2024-02-23 09:58:25 浏览: 22
要使用FFmpeg推送JT1078协议的G711a音频帧数据,可以按照以下步骤进行:
1. 首先,需要初始化FFmpeg的网络模块,可以使用avformat_network_init()函数进行初始化。
2. 创建一个AVFormatContext对象,并设置其输出格式为RTSP,可以使用avformat_alloc_output_context2()函数进行创建。
3. 添加RTSP服务器的地址和端口,可以使用avformat_new_stream()函数创建音频流,设置音频流的编码格式、采样率、通道数等参数。
4. 打开音频编码器,可以使用avcodec_find_encoder()函数查找合适的音频编码器,使用avcodec_open2()函数打开编码器。
5. 将G711a音频帧数据进行编码,可以使用avcodec_send_frame()函数发送音频帧数据,使用avcodec_receive_packet()函数接收编码后的音频包数据。
6. 将编码后的音频包数据进行封装并推送至RTSP服务器,可以使用av_write_frame()函数将音频包写入输出流。
7. 最后,释放资源并关闭输出流,可以使用av_write_trailer()函数进行输出流的封装并释放资源,使用avformat_free_context()函数释放AVFormatContext对象。
具体的代码实现可以参考以下示例:
```
#include <iostream>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <pthread.h>
extern "C" {
#include "libavformat/avformat.h"
#include "libavcodec/avcodec.h"
}
// RTSP服务器地址
#define RTSP_URL "rtsp://127.0.0.1:8554/live.sdp"
int main(int argc, char* argv[]) {
// 初始化网络模块
avformat_network_init();
// 创建AVFormatContext对象
AVFormatContext* out_fmt_ctx = NULL;
if (avformat_alloc_output_context2(&out_fmt_ctx, NULL, "rtsp", RTSP_URL) < 0) {
std::cout << "avformat_alloc_output_context2 failed" << std::endl;
return -1;
}
// 添加音频流
AVStream* audio_stream = avformat_new_stream(out_fmt_ctx, NULL);
if (audio_stream == NULL) {
std::cout << "avformat_new_stream failed" << std::endl;
return -1;
}
audio_stream->codecpar->codec_type = AVMEDIA_TYPE_AUDIO;
audio_stream->codecpar->codec_id = AV_CODEC_ID_PCM_ALAW;
audio_stream->codecpar->sample_rate = 8000;
audio_stream->codecpar->channels = 1;
// 查找音频编码器
AVCodec* audio_codec = avcodec_find_encoder(audio_stream->codecpar->codec_id);
if (audio_codec == NULL) {
std::cout << "avcodec_find_encoder failed" << std::endl;
return -1;
}
// 打开音频编码器
AVCodecContext* audio_codec_ctx = avcodec_alloc_context3(audio_codec);
if (avcodec_open2(audio_codec_ctx, audio_codec, NULL) < 0) {
std::cout << "avcodec_open2 failed" << std::endl;
return -1;
}
// 创建音频帧
AVFrame* audio_frame = av_frame_alloc();
audio_frame->nb_samples = 160;
audio_frame->format = audio_codec_ctx->sample_fmt;
av_frame_get_buffer(audio_frame, 0);
// 创建音频包
AVPacket* audio_packet = av_packet_alloc();
av_init_packet(audio_packet);
// 打开输出流
if (avio_open(&out_fmt_ctx->pb, RTSP_URL, AVIO_FLAG_WRITE) < 0) {
std::cout << "avio_open failed" << std::endl;
return -1;
}
// 写入文件头
if (avformat_write_header(out_fmt_ctx, NULL) < 0) {
std::cout << "avformat_write_header failed" << std::endl;
return -1;
}
// 推送音频帧数据
uint8_t audio_data[160];
int ret;
while (true) {
// 从G711a音频流中读取音频帧数据
// ...
// 将音频帧数据填充到音频帧中
memcpy(audio_frame->data[0], audio_data, 160);
// 发送音频帧数据到编码器
ret = avcodec_send_frame(audio_codec_ctx, audio_frame);
if (ret < 0) {
std::cout << "avcodec_send_frame failed" << std::endl;
return -1;
}
// 接收编码后的音频包数据
while (ret >= 0) {
ret = avcodec_receive_packet(audio_codec_ctx, audio_packet);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
break;
}
else if (ret < 0) {
std::cout << "avcodec_receive_packet failed" << std::endl;
return -1;
}
// 写入音频包数据
av_packet_rescale_ts(audio_packet, audio_codec_ctx->time_base, audio_stream->time_base);
audio_packet->stream_index = audio_stream->index;
ret = av_write_frame(out_fmt_ctx, audio_packet);
if (ret < 0) {
std::cout << "av_write_frame failed" << std::endl;
return -1;
}
}
}
// 写入文件尾
av_write_trailer(out_fmt_ctx);
// 释放资源
av_packet_free(&audio_packet);
av_frame_free(&audio_frame);
avcodec_free_context(&audio_codec_ctx);
avformat_free_context(out_fmt_ctx);
return 0;
}
```
相关推荐
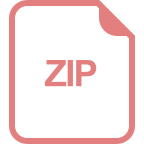














