在一个空间坐标系中,已知沿着Z轴进行切片的多个切片圆的半径和圆心,使用多个切片圆拟合该球体的球心和半径,求C++实现代码
时间: 2023-12-06 18:44:53 浏览: 120
可以使用最小二乘法拟合球心和半径。具体实现步骤如下:
1. 定义一个球体结构体,包含球心坐标和半径:
```cpp
struct Sphere {
double x, y, z; // 球心坐标
double r; // 半径
};
```
2. 定义一个函数,输入为多个切片圆的半径和圆心,输出为拟合得到的球体结构体:
```cpp
Sphere fitSphere(vector<pair<double, tuple<double, double, double>>> circles) {
// 初始化矩阵A和向量b
Eigen::MatrixXd A(circles.size(), 4);
Eigen::VectorXd b(circles.size());
for (int i = 0; i < circles.size(); i++) {
double r = circles[i].first;
double x = get<0>(circles[i].second);
double y = get<1>(circles[i].second);
double z = get<2>(circles[i].second);
A(i, 0) = 2 * x;
A(i, 1) = 2 * y;
A(i, 2) = 2 * z;
A(i, 3) = -1;
b(i) = x * x + y * y + z * z - r * r;
}
// 使用最小二乘法求解
Eigen::VectorXd x = A.jacobiSvd(Eigen::ComputeThinU | Eigen::ComputeThinV).solve(b);
// 将求解结果转换为球体结构体
Sphere sphere;
sphere.x = x(0);
sphere.y = x(1);
sphere.z = x(2);
sphere.r = std::sqrt(x(0) * x(0) + x(1) * x(1) + x(2) * x(2) - x(3));
return sphere;
}
```
3. 调用上述函数进行拟合:
```cpp
vector<pair<double, tuple<double, double, double>>> circles; // 切片圆的半径和圆心
// 添加切片圆
circles.push_back(make_pair(1.0, make_tuple(0.0, 0.0, 0.0)));
circles.push_back(make_pair(2.0, make_tuple(0.0, 0.0, 1.0)));
circles.push_back(make_pair(3.0, make_tuple(0.0, 0.0, 2.0)));
// 拟合球体
Sphere sphere = fitSphere(circles);
// 输出结果
cout << "Sphere center: (" << sphere.x << ", " << sphere.y << ", " << sphere.z << ")" << endl;
cout << "Sphere radius: " << sphere.r << endl;
```
注意:上述代码使用了Eigen库进行矩阵计算,需要事先安装该库。
阅读全文
相关推荐
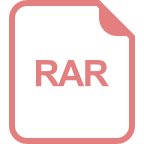
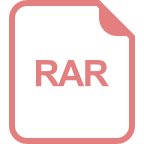
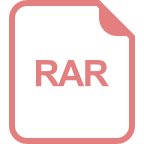
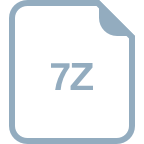
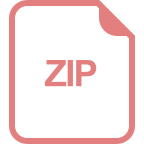
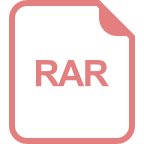
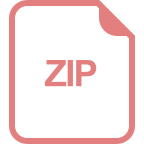
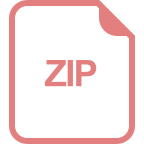
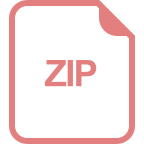
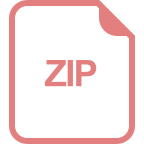
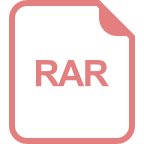
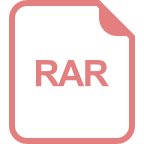