import org.springframework.beans.factory.annotation.Value;
时间: 2024-05-06 14:12:47 浏览: 164
org.springframework.beans.factory.annotation.Value是Spring框架中的一个注解,用于将配置文件中的属性值注入到Java类中的字段或方法参数上。
使用@Value注解可以实现以下功能:
1. 将配置文件中的属性值注入到Java类的字段上,例如:
```
@Value("${property.key}")
private String propertyValue;
```
这样就可以将配置文件中名为"property.key"的属性值注入到propertyValue字段上。
2. 将配置文件中的属性值注入到方法参数上,例如:
```
@RequestMapping("/example")
public String exampleMethod(@Value("${property.key}") String propertyValue) {
// 方法体
}
```
这样就可以将配置文件中名为"property.key"的属性值注入到exampleMethod方法的propertyValue参数上。
3. 支持SpEL表达式,可以在@Value注解中使用SpEL表达式来动态获取属性值,例如:
```
@Value("#{systemProperties['java.home']}")
private String javaHome;
```
这样就可以获取系统属性"java.home"的值并注入到javaHome字段上。
阅读全文
相关推荐
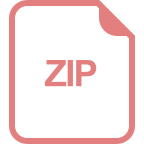
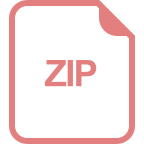















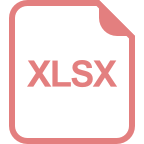
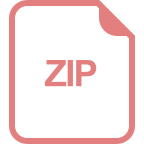