package com.didispace.chapter13; import lombok.extern.slf4j.Slf4j; import org.springframework.beans.factory.annotation.Value; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; @SpringBootApplication public class Chapter13Application { public static void main(String[] args) { SpringApplication.run(Chapter13Application.class, args); } @Slf4j @RestController static class HelloController { @Value("${db:}") private String db; @Value("${mq:}") private String mq; @RequestMapping("/") public String index() { log.info("db:" + db); log.info("mq:" + mq); return ""; } } }
时间: 2024-04-16 16:26:01 浏览: 114
这段代码是一个使用Spring Boot开发的应用程序,与之前的代码几乎相同。主要的区别是使用了lombok的@Slf4j注解替换了原来的@Log4j注解。@Slf4j注解可以自动为HelloController类生成一个名为log的日志对象,从而可以在代码中直接使用log对象进行日志记录。其他的代码逻辑和之前的版本一致。
相关问题
package com.didispace.chapter13; import lombok.extern.log4j.Log4j; import lombok.extern.slf4j.Slf4j; import org.springframework.beans.factory.annotation.Value; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; @SpringBootApplication public class Chapter13Application { public static void main(String[] args) { SpringApplication.run(Chapter13Application.class, args); } @Slf4j @RestController static class HelloController { @Value("${db:}") private String db; @Value("${mq:}") private String mq; @RequestMapping("/") @Log4j public String index() { log.info("db:" + db); log.info("mq:" + mq); return ""; } } }
这是一个使用Spring Boot开发的应用程序,主要包含了一个HelloController类。在该类中,通过@RestController注解将其标记为控制器,并使用@RequestMapping注解将index()方法映射到根路径"/"。在index()方法中,使用@Value注解将配置文件中的db和mq属性注入到对应的变量中,并使用lombok的@Slf4j注解添加日志功能。最后,通过log.info()方法打印db和mq的值。这个应用程序的主要功能是根据配置文件中的属性值,在访问根路径"/"时打印出db和mq的值。
package com.jy.common.netty.strategy; import com.jy.common.netty.protobuf.Message; import io.netty.channel.ChannelHandlerContext; import lombok.extern.slf4j.Slf4j; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.context.annotation.Bean; import org.springframework.stereotype.Component; import javax.annotation.PostConstruct; import java.util.List; import java.util.Map; import java.util.stream.Collectors; @Component public class StrategyFactory { @Autowired private List<ChannelReadStrategy> channelReadStrategies; public void channelRead(ChannelHandlerContext ctx, Object t){ Message message= (Message) t; for(ChannelReadStrategy strategy : channelReadStrategies){ if(strategy.getCommandType()==message.getCmd()){ strategy.channelRead(ctx,t); } } } } 优化洗这段代码
1. 使用 @Slf4j 注解替换掉日志的手动声明,简化代码。
2. 将 @PostConstruct 注解添加到 channelReadStrategies 的初始化方法上,确保在依赖注入完成后进行初始化。
3. 将 for 循环替换为 Java 8 的 Stream API,使用 filter 和 findFirst 方法来查找符合条件的 ChannelReadStrategy 对象,并调用其 channelRead 方法。
4. 将 StrategyFactory 类的 Bean 声明移动到配置类中,使用 @Configuration 和 @Bean 注解来声明该 Bean。
具体代码如下:
```java
package com.jy.common.netty.strategy;
import com.jy.common.netty.protobuf.Message;
import io.netty.channel.ChannelHandlerContext;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Configuration;
import javax.annotation.PostConstruct;
import java.util.List;
@Configuration
@Slf4j
public class StrategyFactory {
@Autowired
private List<ChannelReadStrategy> channelReadStrategies;
@PostConstruct
public void init() {
log.info("Initialized {} channel read strategies", channelReadStrategies.size());
}
public void channelRead(ChannelHandlerContext ctx, Object t) {
Message message = (Message) t;
channelReadStrategies.stream()
.filter(strategy -> strategy.getCommandType() == message.getCmd())
.findFirst()
.ifPresent(strategy -> strategy.channelRead(ctx, t));
}
}
```
阅读全文
相关推荐
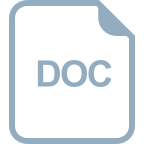
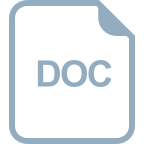
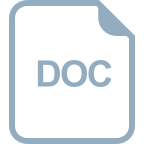



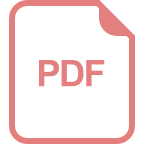
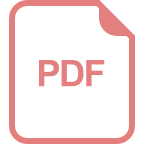
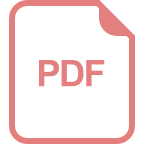
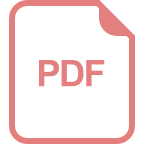
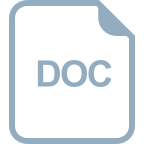
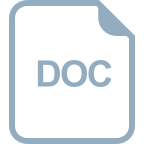
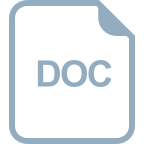
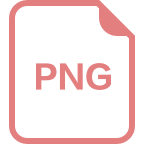