用java写一份图书管理系统
时间: 2023-11-17 20:07:12 浏览: 62
好的,下面是一个简单的图书管理系统的Java代码示例。
```
import java.util.ArrayList;
import java.util.Scanner;
public class BookManagementSystem {
private ArrayList<Book> bookList;
public BookManagementSystem() {
bookList = new ArrayList<Book>();
}
public void addBook(Book book) {
bookList.add(book);
}
public Book findBook(String title) {
for (Book book : bookList) {
if (book.getTitle().equals(title)) {
return book;
}
}
return null;
}
public ArrayList<Book> getBookList() {
return bookList;
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
BookManagementSystem bookManagementSystem = new BookManagementSystem();
while (true) {
System.out.println("请选择操作:");
System.out.println("1.添加图书");
System.out.println("2.查找图书");
System.out.println("3.退出");
int choice = scanner.nextInt();
if (choice == 1) {
System.out.println("请输入图书的标题和作者:");
String title = scanner.next();
String author = scanner.next();
Book book = new Book(title, author);
bookManagementSystem.addBook(book);
System.out.println("添加成功!");
} else if (choice == 2) {
System.out.println("请输入要查找的图书的标题:");
String title = scanner.next();
Book book = bookManagementSystem.findBook(title);
if (book == null) {
System.out.println("未找到该图书!");
} else {
System.out.println(book);
}
} else if (choice == 3) {
System.out.println("感谢使用图书管理系统!");
break;
} else {
System.out.println("输入错误,请重新输入!");
}
}
}
}
class Book {
private String title;
private String author;
public Book(String title, String author) {
this.title = title;
this.author = author;
}
public String getTitle() {
return title;
}
public String getAuthor() {
return author;
}
public String toString() {
return "《" + title + "》 作者:" + author;
}
}
```
该程序实现了基本的图书管理功能,包括添加图书和查找图书。用户可以通过控制台输入来进行操作。需要注意的是,该示例只是一个简单的示例,实际应用中可能需要更加完善的功能和界面设计。
阅读全文
相关推荐






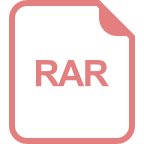
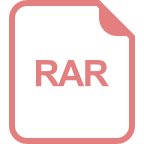









