帮我用C++写一份关于图书管理系统的代码
时间: 2024-03-03 21:49:24 浏览: 26
好的,以下是一个简单的图书管理系统的C++代码示例,实现了基本的图书信息录入、查询、修改和删除功能,供您参考:
```c++
#include <iostream>
#include <string>
using namespace std;
// 图书类
class Book {
private:
string name; // 书名
string author; // 作者
string publisher; // 出版社
string ISBN; // ISBN号
double price; // 价格
public:
// 构造函数
Book(string n, string a, string p, string i, double pr) {
name = n;
author = a;
publisher = p;
ISBN = i;
price = pr;
}
// 获取书名
string getName() {
return name;
}
// 获取作者
string getAuthor() {
return author;
}
// 获取出版社
string getPublisher() {
return publisher;
}
// 获取ISBN号
string getISBN() {
return ISBN;
}
// 获取价格
double getPrice() {
return price;
}
// 修改价格
void setPrice(double pr) {
price = pr;
}
// 显示图书信息
void display() {
cout << "书名:" << name << endl;
cout << "作者:" << author << endl;
cout << "出版社:" << publisher << endl;
cout << "ISBN号:" << ISBN << endl;
cout << "价格:" << price << endl;
cout << endl;
}
};
// 图书管理类
class BookManager {
private:
Book *books[100]; // 图书数组,最多存放100本书
int count; // 当前存储的书籍数量
public:
// 构造函数
BookManager() {
count = 0;
}
// 添加图书
void addBook(Book *book) {
if (count < 100) {
books[count++] = book;
cout << "添加成功!" << endl;
} else {
cout << "添加失败,已经达到最大存储量!" << endl;
}
}
// 根据书名查找图书
void searchByName(string name) {
for (int i = 0; i < count; i++) {
if (books[i]->getName() == name) {
books[i]->display();
return;
}
}
cout << "未找到该书籍!" << endl;
}
// 根据作者查找图书
void searchByAuthor(string author) {
for (int i = 0; i < count; i++) {
if (books[i]->getAuthor() == author) {
books[i]->display();
}
}
}
// 根据ISBN号查找图书
void searchByISBN(string ISBN) {
for (int i = 0; i < count; i++) {
if (books[i]->getISBN() == ISBN) {
books[i]->display();
return;
}
}
cout << "未找到该书籍!" << endl;
}
// 修改图书价格
void updatePrice(string ISBN, double price) {
for (int i = 0; i < count; i++) {
if (books[i]->getISBN() == ISBN) {
books[i]->setPrice(price);
cout << "修改成功!" << endl;
return;
}
}
cout << "未找到该书籍!" << endl;
}
// 删除图书
void deleteBook(string ISBN) {
for (int i = 0; i < count; i++) {
if (books[i]->getISBN() == ISBN) {
for (int j = i; j < count - 1; j++) {
books[j] = books[j + 1];
}
count--;
cout << "删除成功!" << endl;
return;
}
}
cout << "未找到该书籍!" << endl;
}
// 显示所有图书信息
void displayAll() {
for (int i = 0; i < count; i++) {
books[i]->display();
}
}
};
int main() {
BookManager manager; // 图书管理对象
// 添加图书
Book *book1 = new Book("C++程序设计", "谭浩强", "清华大学出版社", "9787302354172", 49.8);
manager.addBook(book1);
Book *book2 = new Book("Java编程思想", "Bruce Eckel", "机械工业出版社", "9787111407010", 99.0);
manager.addBook(book2);
// 查询图书
manager.searchByName("C++程序设计");
// 修改图书价格
manager.updatePrice("9787111407010", 88.0);
// 删除图书
manager.deleteBook("9787302354172");
// 显示所有图书信息
manager.displayAll();
return 0;
}
```
以上代码仅作为参考,实际项目中需要根据具体需求进行修改和完善。
相关推荐
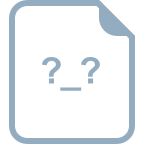
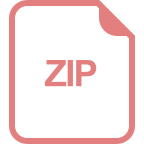
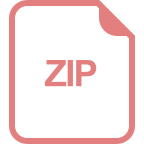














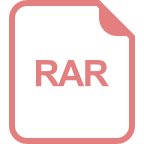