帮我用C++写一份较为完整的图书管理系统,能在控制台进行操作,需要基类,派生类,要能进行数据的保存和读取。
时间: 2024-03-04 21:48:08 浏览: 22
好的,以下是一份C++的基于控制台的图书管理系统代码:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
using namespace std;
// 定义基类Book
class Book {
protected:
string title; // 书名
string author; // 作者
int year; // 出版年份
int pages; // 页数
public:
Book(string t, string a, int y, int p) : title(t), author(a), year(y), pages(p) {}
virtual void display() = 0; // 显示图书信息
virtual string getType() = 0; // 获取图书类型
virtual string getInfo() = 0; // 获取图书信息
};
// 定义派生类Fiction
class Fiction : public Book {
private:
string genre; // 小说类型
public:
Fiction(string t, string a, int y, int p, string g) : Book(t, a, y, p), genre(g) {}
void display() {
cout << "小说: " << title << " 作者: " << author << " 出版年份: " << year << " 页数: " << pages << " 类型: " << genre << endl;
}
string getType() {
return "Fiction";
}
string getInfo() {
return title + "," + author + "," + to_string(year) + "," + to_string(pages) + "," + genre;
}
};
// 定义派生类NonFiction
class NonFiction : public Book {
private:
string topic; // 非小说主题
public:
NonFiction(string t, string a, int y, int p, string tp) : Book(t, a, y, p), topic(tp) {}
void display() {
cout << "非小说: " << title << " 作者: " << author << " 出版年份: " << year << " 页数: " << pages << " 主题: " << topic << endl;
}
string getType() {
return "NonFiction";
}
string getInfo() {
return title + "," + author + "," + to_string(year) + "," + to_string(pages) + "," + topic;
}
};
// 定义图书管理系统类
class BookManager {
private:
vector<Book*> books; // 存储图书的容器
public:
BookManager() {
loadBooks(); // 读取之前保存的图书信息
}
// 添加图书
void addBook(Book* book) {
books.push_back(book);
saveBooks(); // 保存图书信息
}
// 显示所有图书
void displayAllBooks() {
for (auto book : books) {
book->display();
}
}
// 根据类型显示图书
void displayBooksByType(string type) {
for (auto book : books) {
if (book->getType() == type) {
book->display();
}
}
}
// 保存图书信息到文件
void saveBooks() {
ofstream file("books.txt");
for (auto book : books) {
file << book->getType() << "," << book->getInfo() << endl;
}
file.close();
}
// 读取文件中的图书信息
void loadBooks() {
ifstream file("books.txt");
if (file) {
string line;
while (getline(file, line)) {
int pos = line.find(",");
string type = line.substr(0, pos);
string info = line.substr(pos + 1);
pos = info.find(",");
string title = info.substr(0, pos);
info = info.substr(pos + 1);
pos = info.find(",");
string author = info.substr(0, pos);
info = info.substr(pos + 1);
pos = info.find(",");
int year = stoi(info.substr(0, pos));
info = info.substr(pos + 1);
pos = info.find(",");
int pages = stoi(info.substr(0, pos));
info = info.substr(pos + 1);
if (type == "Fiction") {
pos = info.find(",");
string genre = info.substr(0, pos);
addBook(new Fiction(title, author, year, pages, genre));
}
else if (type == "NonFiction") {
pos = info.find(",");
string topic = info.substr(0, pos);
addBook(new NonFiction(title, author, year, pages, topic));
}
}
}
file.close();
}
};
int main() {
BookManager manager;
while (true) {
cout << "请选择操作: " << endl;
cout << "1. 添加小说" << endl;
cout << "2. 添加非小说" << endl;
cout << "3. 显示所有图书" << endl;
cout << "4. 显示小说" << endl;
cout << "5. 显示非小说" << endl;
cout << "6. 退出" << endl;
int choice;
cin >> choice;
if (choice == 1) {
string title, author, genre;
int year, pages;
cout << "请输入小说信息: " << endl;
cout << "书名: ";
cin >> title;
cout << "作者: ";
cin >> author;
cout << "出版年份: ";
cin >> year;
cout << "页数: ";
cin >> pages;
cout << "类型: ";
cin >> genre;
manager.addBook(new Fiction(title, author, year, pages, genre));
}
else if (choice == 2) {
string title, author, topic;
int year, pages;
cout << "请输入非小说信息: " << endl;
cout << "书名: ";
cin >> title;
cout << "作者: ";
cin >> author;
cout << "出版年份: ";
cin >> year;
cout << "页数: ";
cin >> pages;
cout << "主题: ";
cin >> topic;
manager.addBook(new NonFiction(title, author, year, pages, topic));
}
else if (choice == 3) {
manager.displayAllBooks();
}
else if (choice == 4) {
manager.displayBooksByType("Fiction");
}
else if (choice == 5) {
manager.displayBooksByType("NonFiction");
}
else if (choice == 6) {
break;
}
else {
cout << "无效的选择,请重新输入!" << endl;
}
}
return 0;
}
```
该代码实现了基类Book和派生类Fiction、NonFiction,以及图书管理系统类BookManager,可以在控制台中添加、显示图书,并且能够将图书信息保存到文件中,下次运行程序时可以读取之前保存的图书信息。
相关推荐
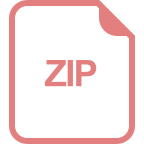
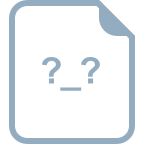
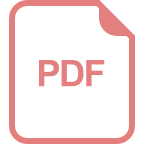











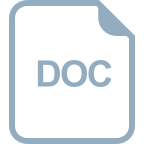
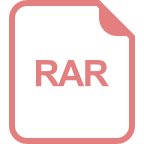
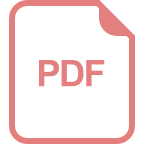
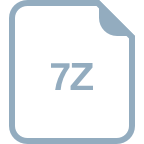