用Java写一份复杂的图书管理系统
时间: 2023-09-30 13:01:51 浏览: 78
一个完整的图书管理系统需要包括图书的分类管理、图书借阅管理、读者管理、图书馆管理等多个方面的功能。下面是一个简单的Java代码示例,包含了图书分类、图书借阅和读者管理三个模块的实现。
```
import java.util.ArrayList;
import java.util.Date;
import java.util.HashMap;
import java.util.Scanner;
public class LibraryManagementSystem {
private HashMap<String, ArrayList<Book>> bookMap;
private ArrayList<BorrowRecord> borrowRecordList;
private ArrayList<Reader> readerList;
public LibraryManagementSystem() {
bookMap = new HashMap<String, ArrayList<Book>>();
borrowRecordList = new ArrayList<BorrowRecord>();
readerList = new ArrayList<Reader>();
}
public void addBook(Book book) {
String category = book.getCategory();
if (!bookMap.containsKey(category)) {
bookMap.put(category, new ArrayList<Book>());
}
bookMap.get(category).add(book);
}
public ArrayList<Book> findBookByCategory(String category) {
if (!bookMap.containsKey(category)) {
return new ArrayList<Book>();
}
return bookMap.get(category);
}
public void borrowBook(Book book, Reader reader) throws Exception {
if (book.getStatus() == Book.Status.BORROWED) {
throw new Exception("该图书已被借出!");
}
book.setStatus(Book.Status.BORROWED);
BorrowRecord borrowRecord = new BorrowRecord(book, reader, new Date());
borrowRecordList.add(borrowRecord);
}
public void returnBook(Book book, Reader reader) throws Exception {
BorrowRecord borrowRecord = null;
for (BorrowRecord record : borrowRecordList) {
if (record.getBook() == book && record.getReader() == reader) {
borrowRecord = record;
break;
}
}
if (borrowRecord == null) {
throw new Exception("未找到该借阅记录!");
}
if (borrowRecord.getReturnDate() != null) {
throw new Exception("该图书已归还!");
}
borrowRecord.setReturnDate(new Date());
book.setStatus(Book.Status.AVAILABLE);
}
public void addReader(Reader reader) {
readerList.add(reader);
}
public Reader findReader(String id) {
for (Reader reader : readerList) {
if (reader.getId().equals(id)) {
return reader;
}
}
return null;
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
LibraryManagementSystem libraryManagementSystem = new LibraryManagementSystem();
while (true) {
System.out.println("请选择操作:");
System.out.println("1.添加图书");
System.out.println("2.查找图书");
System.out.println("3.借出图书");
System.out.println("4.归还图书");
System.out.println("5.添加读者");
System.out.println("6.查找读者");
System.out.println("7.退出");
int choice = scanner.nextInt();
if (choice == 1) {
System.out.println("请输入图书的标题、作者和分类:");
String title = scanner.next();
String author = scanner.next();
String category = scanner.next();
Book book = new Book(title, author, category);
libraryManagementSystem.addBook(book);
System.out.println("添加成功!");
} else if (choice == 2) {
System.out.println("请输入要查找的图书的分类:");
String category = scanner.next();
ArrayList<Book> bookList = libraryManagementSystem.findBookByCategory(category);
System.out.println("找到 " + bookList.size() + " 本图书:");
for (Book book : bookList) {
System.out.println(book);
}
} else if (choice == 3) {
System.out.println("请输入要借阅的图书的标题和作者:");
String title = scanner.next();
String author = scanner.next();
Book book = null;
for (ArrayList<Book> list : libraryManagementSystem.bookMap.values()) {
for (Book b : list) {
if (b.getTitle().equals(title) && b.getAuthor().equals(author)) {
book = b;
break;
}
}
if (book != null) {
break;
}
}
if (book == null) {
System.out.println("未找到该图书!");
continue;
}
System.out.println("请输入借阅人的ID:");
String readerId = scanner.next();
Reader reader = libraryManagementSystem.findReader(readerId);
if (reader == null) {
System.out.println("未找到该读者!");
continue;
}
try {
libraryManagementSystem.borrowBook(book, reader);
System.out.println("借阅成功!");
} catch (Exception e) {
System.out.println(e.getMessage());
}
} else if (choice == 4) {
System.out.println("请输入要归还的图书的标题和作者:");
String title = scanner.next();
String author = scanner.next();
Book book = null;
for (ArrayList<Book> list : libraryManagementSystem.bookMap.values()) {
for (Book b : list) {
if (b.getTitle().equals(title) && b.getAuthor().equals(author)) {
book = b;
break;
}
}
if (book != null) {
break;
}
}
if (book == null) {
System.out.println("未找到该图书!");
continue;
}
System.out.println("请输入归还人的ID:");
String readerId = scanner.next();
Reader reader = libraryManagementSystem.findReader(readerId);
if (reader == null) {
System.out.println("未找到该读者!");
continue;
}
try {
libraryManagementSystem.returnBook(book, reader);
System.out.println("归还成功!");
} catch (Exception e) {
System.out.println(e.getMessage());
}
} else if (choice == 5) {
System.out.println("请输入读者的ID和姓名:");
String id = scanner.next();
String name = scanner.next();
Reader reader = new Reader(id, name);
libraryManagementSystem.addReader(reader);
System.out.println("添加成功!");
} else if (choice == 6) {
System.out.println("请输入要查找的读者的ID:");
String id = scanner.next();
Reader reader = libraryManagementSystem.findReader(id);
if (reader == null) {
System.out.println("未找到该读者!");
} else {
System.out.println(reader);
}
} else if (choice == 7) {
System.out.println("感谢使用图书馆管理系统!");
break;
} else {
System.out.println("输入错误,请重新输入!");
}
}
}
}
class Book {
private String title;
private String author;
private String category;
private Book.Status status;
public Book(String title, String author, String category) {
this.title = title;
this.author = author;
this.category = category;
this.status = Book.Status.AVAILABLE;
}
public String getTitle() {
return title;
}
public String getAuthor() {
return author;
}
public String getCategory() {
return category;
}
public Book.Status getStatus() {
return status;
}
public void setStatus(Book.Status status) {
this.status = status;
}
public String toString() {
return "《" + title + "》 作者:" + author + " 分类:" + category;
}
public enum Status {
AVAILABLE,
BORROWED
}
}
class BorrowRecord {
private Book book;
private Reader reader;
private Date borrowDate;
private Date returnDate;
public BorrowRecord(Book book, Reader reader, Date borrowDate) {
this.book = book;
this.reader = reader;
this.borrowDate = borrowDate;
}
public Book getBook() {
return book;
}
public Reader getReader() {
return reader;
}
public Date getBorrowDate() {
return borrowDate;
}
public Date getReturnDate() {
return returnDate;
}
public void setReturnDate(Date returnDate) {
this.returnDate = returnDate;
}
}
class Reader {
private String id;
private String name;
public Reader(String id, String name) {
this.id = id;
this.name = name;
}
public String getId() {
return id;
}
public String getName() {
return name;
}
public String toString() {
return "读者ID:" + id + " 姓名:" + name;
}
}
```
该程序实现了基本的图书分类、图书借阅和读者管理功能,包括添加图书、查找图书、借出图书、归还图书、添加读者、查找读者等。需要注意的是,该示例只是一个简单的示例,实际应用中可能需要更加完善的功能和界面设计。
阅读全文
相关推荐
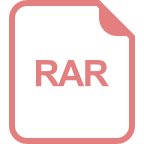






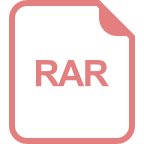
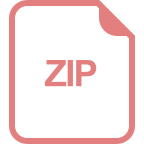
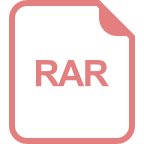
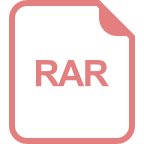
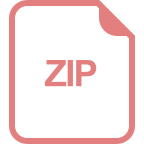
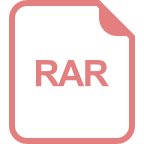
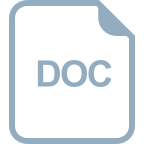
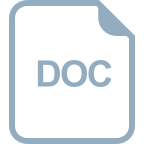
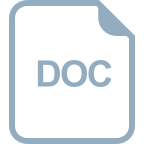
