在部分类别数量稀少的且训练集样本很少情况下,进行14分类的算法,并可视化预测结果以及图Python实现完成代码
时间: 2024-09-25 15:08:33 浏览: 41
在这种情况下,处理少量样本且类别分布不均衡的数据集通常会遇到过拟合的风险。为了降低过拟合并提高泛化能力,可以考虑以下策略:
1. **降维**:尝试使用主成分分析(PCA)或线性判别分析(LDA)等技术减少特征的数量,从而减少模型复杂度。
2. **集成学习**:例如使用随机森林(Random Forest)或梯度提升机(Gradient Boosting Machine),它们能结合多个弱分类器形成强分类器,缓解过拟合。
3. **平衡类别**:如果类别不平衡严重,可以尝试欠采样、过采样或生成合成样本(如SMOTE)来改善数据分布。
4. **正则化**:对于一些机器学习模型,比如SVM,可以增加正则化参数来控制复杂度。
5. **早停**:在训练过程中监控验证集的表现,一旦验证集性能不再提升就停止训练,防止过拟合。
关于代码实现,这里给出一个简单的示例,使用sklearn库中的`RandomForestClassifier`和`matplotlib`库进行可视化。假设已经对数据进行了预处理:
```python
import numpy as np
import pandas as pd
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.preprocessing import StandardScaler
import matplotlib.pyplot as plt
# 创建模拟数据,这里假设只有14个类别
X, y = make_classification(n_samples=100, n_features=20, n_classes=14, class_sep=2, random_state=42)
# 数据标准化
scaler = StandardScaler()
X_scaled = scaler.fit_transform(X)
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X_scaled, y, test_size=0.2, random_state=42)
# 使用随机森林分类器
clf = RandomForestClassifier(random_state=42)
clf.fit(X_train, y_train)
# 预测
y_pred = clf.predict(X_test)
# 混淆矩阵可视化
conf_matrix = confusion_matrix(y_test, y_pred)
plt.figure(figsize=(12, 10))
plt.imshow(conf_matrix, cmap='Blues', interpolation='nearest')
plt.colorbar()
tick_marks = np.arange(len(np.unique(y)))
plt.xticks(tick_marks, np.unique(y), rotation=45)
plt.yticks(tick_marks, np.unique(y))
# 计算并打印精度、召回率等
from sklearn.metrics import classification_report
report = classification_report(y_test, y_pred, target_names=np.unique(y), output_dict=True)
# 打印每个类别的性能
for label, metrics in report.items():
print(f"Class {label}:")
print("Precision:", metrics['precision'])
print("Recall:", metrics['recall'])
plt.xlabel('Predicted')
plt.ylabel('True')
plt.title('Confusion Matrix')
plt.show()
```
注意,这个示例是一个基础演示,实际情况可能需要根据数据的具体情况进行适当的修改和优化。特别是当类别极度不平衡时,可能还需要针对类别调整阈值或者使用其他策略。
阅读全文
相关推荐
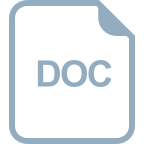
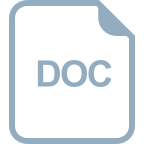
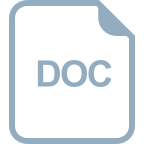


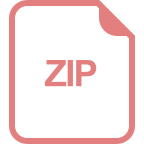
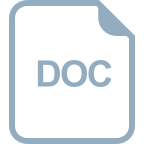
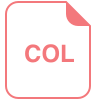
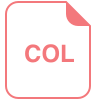
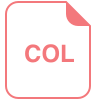
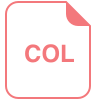
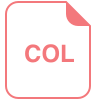
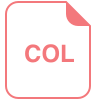
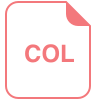
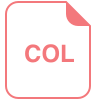
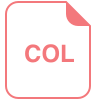

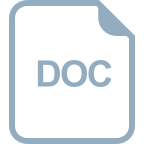
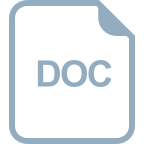