windows下qt使用zlib压缩文件夹,解压缩
时间: 2023-08-21 08:05:00 浏览: 74
在Windows下使用Qt进行文件夹压缩和解压缩,可以使用zlib库进行实现。以下是实现的基本步骤:
1. 下载zlib库并将其添加到Qt项目中。
2. 使用zlib库的函数进行文件夹压缩,代码示例:
```cpp
#include <zlib.h>
// 压缩文件夹
bool compressFolder(QString folderPath, QString zipFilePath) {
QDir dir(folderPath);
if (!dir.exists()) {
qDebug() << "Folder not exists!";
return false;
}
QFile zipFile(zipFilePath);
if (!zipFile.open(QIODevice::WriteOnly)) {
qDebug() << "Failed to create zip file!";
return false;
}
// 初始化zlib stream
z_stream stream;
stream.zalloc = Z_NULL;
stream.zfree = Z_NULL;
stream.opaque = Z_NULL;
deflateInit(&stream, Z_DEFAULT_COMPRESSION);
// 遍历文件夹下所有文件进行压缩
QList<QFileInfo> fileInfoList = dir.entryInfoList(QDir::Files | QDir::Dirs | QDir::NoDotAndDotDot);
foreach (QFileInfo fileInfo, fileInfoList) {
if (fileInfo.isDir()) {
QString subFolderPath = fileInfo.filePath();
QString subZipFilePath = zipFilePath + "/" + fileInfo.fileName() + ".zip";
compressFolder(subFolderPath, subZipFilePath);
} else {
QFile file(fileInfo.filePath());
if (!file.open(QIODevice::ReadOnly)) {
qDebug() << "Failed to open file: " << fileInfo.filePath();
continue;
}
QByteArray data = file.readAll();
QByteArray compressedData(data.size(), Qt::Uninitialized);
stream.avail_in = data.size();
stream.next_in = (Bytef *)data.data();
stream.avail_out = compressedData.size();
stream.next_out = (Bytef *)compressedData.data();
deflate(&stream, Z_FINISH);
compressedData.resize(stream.total_out);
zipFile.write(compressedData);
}
}
// 完成压缩
deflateEnd(&stream);
zipFile.close();
return true;
}
```
3. 使用zlib库的函数进行文件夹解压缩,代码示例:
```cpp
#include <zlib.h>
// 解压文件夹
bool decompressFolder(QString zipFilePath, QString folderPath) {
QFile zipFile(zipFilePath);
if (!zipFile.open(QIODevice::ReadOnly)) {
qDebug() << "Failed to open zip file!";
return false;
}
QDir dir(folderPath);
if (!dir.exists()) {
dir.mkpath(".");
}
// 初始化zlib stream
z_stream stream;
stream.zalloc = Z_NULL;
stream.zfree = Z_NULL;
stream.opaque = Z_NULL;
inflateInit(&stream);
// 逐个解压文件
QByteArray buffer(1024 * 1024, Qt::Uninitialized);
while (!zipFile.atEnd()) {
QByteArray compressedData = zipFile.read(buffer.size());
buffer.resize(compressedData.size() * 2);
stream.avail_in = compressedData.size();
stream.next_in = (Bytef *)compressedData.data();
stream.avail_out = buffer.size();
stream.next_out = (Bytef *)buffer.data();
int result = inflate(&stream, Z_SYNC_FLUSH);
if (result != Z_OK && result != Z_STREAM_END) {
qDebug() << "Failed to decompress data!";
inflateEnd(&stream);
return false;
}
buffer.resize(buffer.size() - stream.avail_out);
QString fileName = QFileInfo(zipFilePath).baseName();
QFile file(folderPath + "/" + fileName);
if (!file.open(QIODevice::WriteOnly | QIODevice::Append)) {
qDebug() << "Failed to create file: " << folderPath + "/" + fileName;
continue;
}
file.write(buffer);
file.close();
}
// 完成解压缩
inflateEnd(&stream);
zipFile.close();
return true;
}
```
这样,就可以使用zlib库进行文件夹压缩和解压缩了。
相关推荐
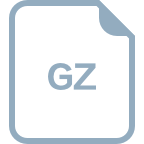
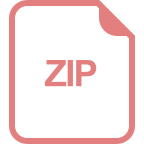











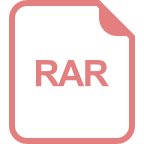
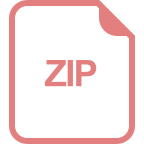